粒子群算法实现旅行商问题代码
时间: 2023-07-14 07:14:20 浏览: 106
以下是使用粒子群算法(PSO)解决旅行商问题(TSP)的 Python 代码示例:
```python
import random
import math
# 城市坐标
city_location = [
[41, 94], [37, 84], [54, 67], [25, 62], [7, 64],
[2, 99], [68, 58], [71, 44], [54, 62], [83, 69]
]
# 城市数量
city_num = 10
# 种群数量
pop_size = 50
# 迭代次数
max_iter = 500
# 学习因子
w = 0.6
# 加速因子
c1 = 1.5
c2 = 1.5
# 初始化粒子群
class Particle:
def __init__(self):
self.position = random.sample(range(city_num), city_num)
self.velocity = [0] * city_num
self.fitness = self.cal_fitness()
# 计算适应度函数
def cal_fitness(self):
distance = 0
for i in range(city_num - 1):
distance += math.sqrt((city_location[self.position[i]][0] - city_location[self.position[i+1]][0]) ** 2
+ (city_location[self.position[i]][1] - city_location[self.position[i+1]][1]) ** 2)
distance += math.sqrt((city_location[self.position[0]][0] - city_location[self.position[city_num-1]][0]) ** 2
+ (city_location[self.position[0]][1] - city_location[self.position[city_num-1]][1]) ** 2)
return 1 / distance
class PSO:
def __init__(self):
self.gbest = Particle()
self.particles = [Particle() for i in range(pop_size)]
# 更新粒子群
def update(self):
for p in self.particles:
for i in range(city_num):
p.velocity[i] = w * p.velocity[i] \
+ c1 * random.random() * (p.best.position[i] - p.position[i]) \
+ c2 * random.random() * (self.gbest.position[i] - p.position[i])
p.position[i] = int(p.position[i] + p.velocity[i] % city_num)
p.fitness = p.cal_fitness()
if p.fitness > p.best.fitness:
p.best.position = p.position[:]
p.best.fitness = p.fitness
if p.fitness > self.gbest.fitness:
self.gbest.position = p.position[:]
self.gbest.fitness = p.fitness
# 运行PSO算法
def run(self):
for i in range(max_iter):
self.update()
print("Iteration %d: Best Fitness = %f" % (i, self.gbest.fitness))
if __name__ == '__main__':
pso = PSO()
pso.run()
```
在上面的代码中,`city_location` 是城市坐标列表,`city_num` 是城市数量,`pop_size` 是种群数量,`max_iter` 是迭代次数,`w`、`c1` 和 `c2` 分别是学习因子和加速因子。
`Particle` 类表示一个粒子,其中 `position` 是当前粒子的位置,`velocity` 是当前粒子的速度,`fitness` 是当前粒子的适应度。
`PSO` 类表示整个粒子群,其中 `gbest` 是全局最优解,`particles` 是所有粒子的列表。
在 `update()` 方法中,首先更新每个粒子的速度和位置,然后计算每个粒子的适应度,更新每个粒子的个体最优解和全局最优解。
在 `run()` 方法中,运行整个 PSO 算法,输出每次迭代后的最优解。
阅读全文
相关推荐
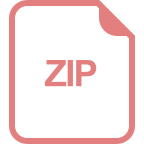
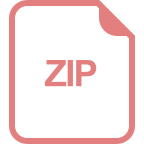
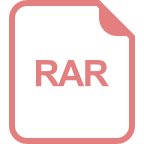
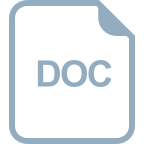
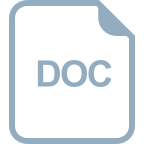
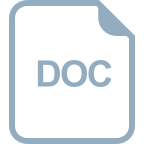
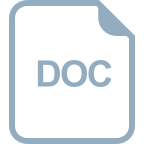


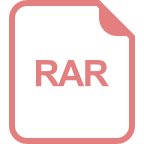
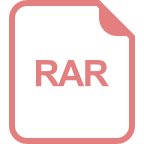
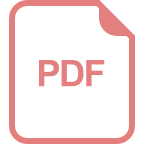
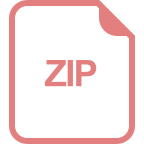
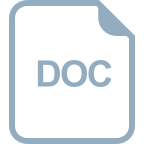
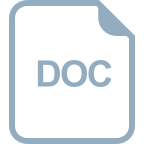