用java语言设计一个基于TCP的文件上传功能,将文件从客户端上传到指定服务器。 并实现文件传输完成后服务器给客户端发送“文件上传成功”的信息。
时间: 2023-12-19 13:04:51 浏览: 73
好的,这个问题我可以回答。以下是基于Java语言设计基于TCP协议的文件上传功能的步骤:
1. 创建客户端和服务器端的Socket连接。
客户端可以使用Socket类进行连接,服务器端可以使用ServerSocket类进行监听。
2. 客户端将文件读取为字节数组并发送到服务器。
可以使用FileInputStream类将文件读取为字节数组,然后使用OutputStream类将字节数组发送到服务器。
3. 服务器接收到字节数组并将其写入文件。
可以使用FileOutputStream类将接收到的字节数组写入文件。
4. 服务器发送“文件上传成功”信息给客户端。
可以使用OutputStream类将“文件上传成功”信息发送到客户端。
5. 客户端接收到“文件上传成功”信息并关闭连接。
可以使用InputStream类接收“文件上传成功”信息,然后关闭连接。
以下是一个简单的实现示例:
客户端代码:
```
import java.io.*;
import java.net.*;
public class FileClient {
public static void main(String[] args) throws Exception {
Socket socket = new Socket("localhost", 8888);
File file = new File("test.txt");
byte[] buffer = new byte[(int)file.length()];
FileInputStream fis = new FileInputStream(file);
fis.read(buffer);
OutputStream os = socket.getOutputStream();
os.write(buffer);
fis.close();
os.close();
InputStream is = socket.getInputStream();
byte[] responseBuffer = new byte[1024];
is.read(responseBuffer);
String response = new String(responseBuffer).trim();
System.out.println(response);
is.close();
socket.close();
}
}
```
服务器端代码:
```
import java.io.*;
import java.net.*;
public class FileServer {
public static void main(String[] args) throws Exception {
ServerSocket serverSocket = new ServerSocket(8888);
Socket socket = serverSocket.accept();
InputStream is = socket.getInputStream();
byte[] buffer = new byte[1024];
ByteArrayOutputStream bos = new ByteArrayOutputStream();
int len;
while ((len = is.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
byte[] data = bos.toByteArray();
FileOutputStream fos = new FileOutputStream("test-copy.txt");
fos.write(data);
fos.close();
OutputStream os = socket.getOutputStream();
os.write("文件上传成功".getBytes("UTF-8"));
os.close();
is.close();
socket.close();
serverSocket.close();
}
}
```
注意:以上示例代码仅供参考,实际应用中需要对异常进行处理,并进行更严谨的验证和测试。
阅读全文
相关推荐
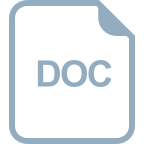
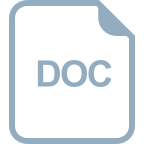
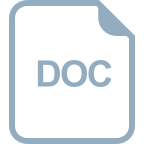



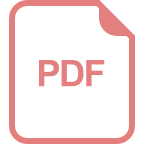
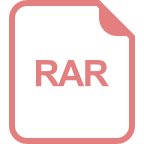
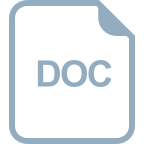
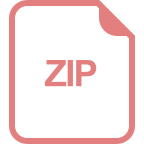
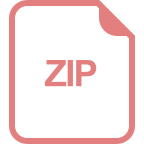
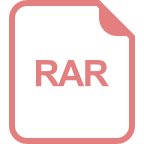
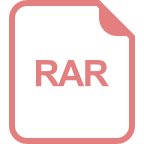
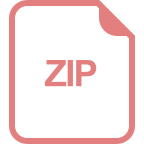
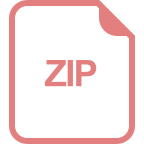
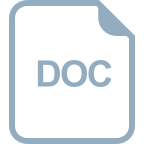
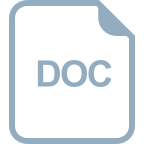
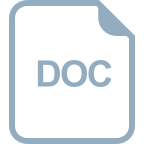
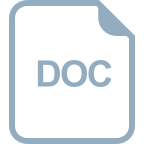