用js和html、css写一个二叉树的深度优先遍历
时间: 2024-05-11 15:14:19 浏览: 7
HTML:
```html
<!DOCTYPE html>
<html>
<head>
<title>二叉树深度优先遍历</title>
<style type="text/css">
.node {
height: 50px;
width: 50px;
background-color: #ccc;
border-radius: 50%;
display: flex;
align-items: center;
justify-content: center;
font-size: 20px;
font-weight: bold;
color: #fff;
}
.tree {
display: flex;
flex-wrap: wrap;
justify-content: center;
margin-top: 50px;
}
.line {
height: 20px;
width: 1px;
background-color: #ccc;
position: absolute;
left: 50%;
transform: translateX(-50%);
z-index: -1;
}
.line.vertical {
height: 50px;
width: 20px;
transform: translate(-50%, -50%);
}
.line.horizontal {
height: 1px;
width: 50px;
transform: translate(-50%, -50%);
}
</style>
</head>
<body>
<div class="tree">
<div class="node" id="node-1">1</div>
<div class="line vertical" id="line-1"></div>
<div class="node" id="node-2">2</div>
<div class="line vertical" id="line-2"></div>
<div class="node" id="node-3">3</div>
<div class="line horizontal" id="line-3"></div>
<div class="line vertical" id="line-4"></div>
<div class="node" id="node-4">4</div>
<div class="line vertical" id="line-5"></div>
<div class="node" id="node-5">5</div>
<div class="line horizontal" id="line-6"></div>
<div class="line vertical" id="line-7"></div>
<div class="node" id="node-6">6</div>
<div class="line vertical" id="line-8"></div>
<div class="node" id="node-7">7</div>
<div class="line vertical" id="line-9"></div>
<div class="node" id="node-8">8</div>
</div>
<script type="text/javascript" src="script.js"></script>
</body>
</html>
```
JS:
```javascript
const nodeList = document.querySelectorAll('.node');
const lineList = document.querySelectorAll('.line');
// 二叉树结构
const tree = {
value: 1,
left: {
value: 2,
left: {
value: 4,
left: null,
right: null
},
right: {
value: 5,
left: null,
right: null
}
},
right: {
value: 3,
left: {
value: 6,
left: null,
right: null
},
right: {
value: 7,
left: null,
right: {
value: 8,
left: null,
right: null
}
}
}
};
// 深度优先遍历
function dfs(node) {
if (node) {
console.log(node.value); // 打印节点的值
dfs(node.left); // 递归遍历左子树
dfs(node.right); // 递归遍历右子树
}
}
dfs(tree); // 从根节点开始遍历
// 显示遍历过程
let index = 0;
function dfsWithAnimation(node) {
if (node) {
setTimeout(() => {
nodeList[index].style.backgroundColor = '#ff0000'; // 用红色表示当前节点
if (node.left) {
// 左子树存在,画一条垂直线连接当前节点和左子节点
lineList[index * 2].style.backgroundColor = '#ff0000';
lineList[index * 2].style.height = '50px';
lineList[index * 2].style.transform = 'translate(-50%, 0)';
lineList[index * 2].style.zIndex = '1';
lineList[index * 2].style.transition = 'height 0.5s, transform 0.5s';
}
if (node.right) {
// 右子树存在,画一条垂直线连接当前节点和右子节点
lineList[index * 2 + 1].style.backgroundColor = '#ff0000';
lineList[index * 2 + 1].style.height = '50px';
lineList[index * 2 + 1].style.transform = 'translate(-50%, 0)';
lineList[index * 2 + 1].style.zIndex = '1';
lineList[index * 2 + 1].style.transition = 'height 0.5s, transform 0.5s';
}
index++;
dfsWithAnimation(node.left); // 递归遍历左子树
dfsWithAnimation(node.right); // 递归遍历右子树
nodeList[index - 1].style.backgroundColor = '#ccc'; // 当前节点遍历完成,恢复原来颜色
if (node.left) {
// 左子树遍历完成,恢复原来颜色
lineList[index * 2 - 2].style.backgroundColor = '#ccc';
lineList[index * 2 - 2].style.height = '20px';
lineList[index * 2 - 2].style.transform = 'translate(-50%, -50%)';
lineList[index * 2 - 2].style.zIndex = '-1';
lineList[index * 2 - 2].style.transition = 'height 0.5s, transform 0.5s';
}
if (node.right) {
// 右子树遍历完成,恢复原来颜色
lineList[index * 2 - 1].style.backgroundColor = '#ccc';
lineList[index * 2 - 1].style.height = '20px';
lineList[index * 2 - 1].style.transform = 'translate(-50%, -50%)';
lineList[index * 2 - 1].style.zIndex = '-1';
lineList[index * 2 - 1].style.transition = 'height 0.5s, transform 0.5s';
}
}, 500 * index);
}
}
dfsWithAnimation(tree); // 从根节点开始遍历
```
相关推荐
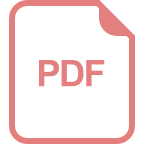
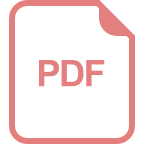















