使用Pyqt6写一个界面,是关于adb push命令的使用,选择本地文件,push到Android系统的指定目录下
时间: 2024-11-03 12:18:04 浏览: 28
要使用PyQt6创建一个简单的GUI应用程序,用于执行`adb push`命令并将本地文件推送到Android设备的指定目录,你需要做以下几个步骤:
1. 首先,确保已安装了`pyqt6`库(如果还没有安装,可以通过`pip install PyQt6`命令安装)。
2. 导入所需的库和模块:
```python
import os
from PyQt6.QtWidgets import QApplication, QWidget, QVBoxLayout, QLabel, QPushButton, QLineEdit, QFileDialog
from PyQt6.QtCore import Slot
```
3. 创建一个窗口类继承自`QWidget`:
```python
class AdbPushApp(QWidget):
def __init__(self):
super().__init__()
# 初始化UI组件
self.init_ui()
def init_ui(self):
layout = QVBoxLayout()
# 文件选择按钮
self.local_file_button = QPushButton("选择本地文件")
self.local_file_button.clicked.connect(self.select_local_file)
layout.addWidget(self.local_file_button)
# 目录输入框
self.target_directory_label = QLabel("请输入目标目录:")
self.target_directory_input = QLineEdit()
layout.addWidget(self.target_directory_label)
layout.addWidget(self.target_directory_input)
# 执行按钮
self.push_button = QPushButton("推送文件")
self.push_button.clicked.connect(self.push_to_device)
layout.addWidget(self.push_button)
self.setLayout(layout)
```
4. 添加文件选择功能:
```python
@Slot()
def select_local_file(self):
options = QFileDialog.Options()
options |= QFileDialog.DontUseNativeDialog
file_path, _ = QFileDialog.getOpenFileName(self, "选择文件", "", "All Files (*);;Text Files (*.txt)", options=options)
if file_path:
self.local_file_label.setText(f"本地文件: {file_path}")
```
5. 添加推送文件的功能,这里只是一个基础示例,实际可能需要处理`adb`命令执行错误和返回结果:
```python
@Slot()
def push_to_device(self):
local_file = self.local_file_label.text().split(": ")[1] # 获取本地文件路径
target_directory = self.target_directory_input.text() # 获取目标目录
adb_command = f"adb -s <device_id> push {local_file} {target_directory}"
# 实际上需要替换<device_id>为连接的设备ID或动态获取
os.system(adb_command) # 运行adb命令
```
6. 最后,启动应用:
```python
if __name__ == "__main__":
app = QApplication([])
window = AdbPushApp()
window.show()
app.exec()
```
阅读全文
相关推荐
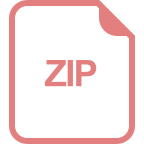
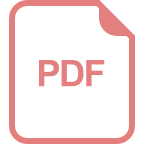
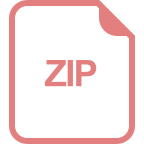
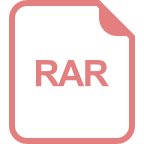
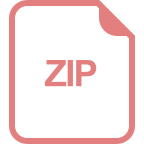
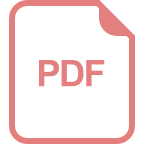
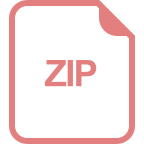
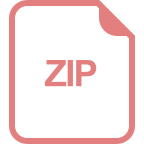
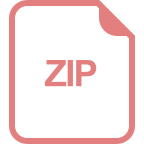
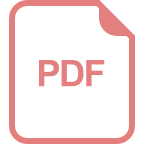
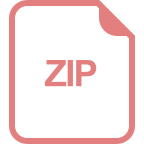
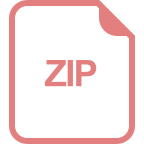
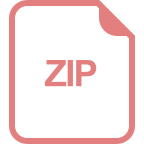
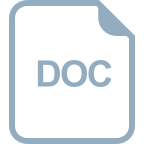
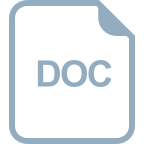