编程实现:线性表顺序存储的基本操作
时间: 2024-10-12 10:11:41 浏览: 39
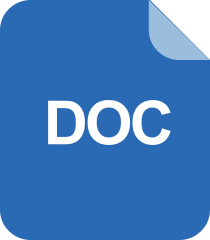
实验一 线性表基本操作的编程实现

线性表顺序存储是指将数据元素按照一定的顺序(如数组的形式)连续地存储在内存中。常见的基本操作包括:
1. **创建(构造)**:初始化一个线性表,可以指定初始长度并全部置零,或者动态分配内存。
```python
def create_list(length):
return [0] * length
```
2. **插入(Insertion)**:在指定位置添加新元素。如果列表已满,需要动态扩展空间。
```python
def insert_at(index, value, lst):
if index < 0 or index > len(lst):
raise IndexError("Index out of range")
lst.append(None) # 或者其他空值,直到找到足够空间
for i in range(len(lst) - 1, index, -1): # shift elements to the right
lst[i] = lst[i - 1]
lst[index] = value
```
3. **删除(Deletion)**:移除指定位置的元素。通常涉及将后续元素向前移动一位。
```python
def delete_at(index, lst):
if index < 0 or index >= len(lst):
raise IndexError("Index out of range")
for i in range(index, len(lst) - 1):
lst[i] = lst[i + 1]
lst.pop() # remove the last element
```
4. **查找(Search)**:在给定值在列表中的存在性。
```python
def search(value, lst):
for i, item in enumerate(lst):
if item == value:
return i
return None # 如果没找到则返回None
```
5. **访问(Traversal)**:遍历整个列表获取所有元素。
```python
def traverse(lst):
for item in lst:
print(item)
```
阅读全文
相关推荐
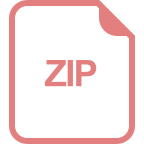
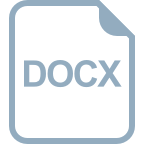
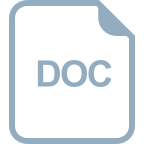
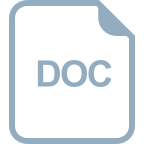
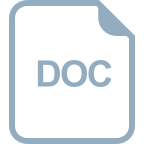
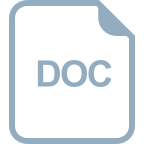
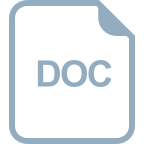
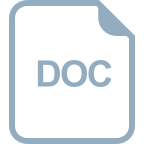
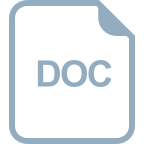
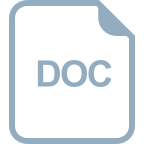
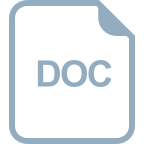
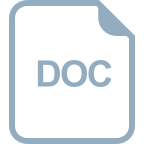
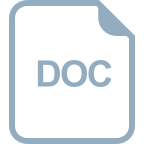
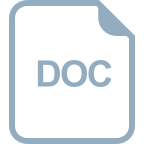
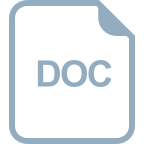
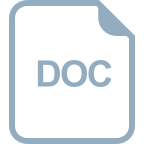
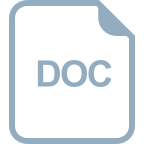
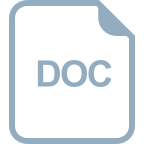