如何用c++读取csv文件里的数据
时间: 2024-04-23 21:27:51 浏览: 162
你可以使用C++标准库中的fstream库来读取CSV文件的数据。下面是一个简单的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
int main() {
std::ifstream file("data.csv");
std::vector<std::vector<std::string>> data;
if (file) {
std::string line;
while (std::getline(file, line)) {
std::vector<std::string> row;
size_t pos = 0;
std::string token;
while ((pos = line.find(',')) != std::string::npos) {
token = line.substr(0, pos);
row.push_back(token);
line.erase(0, pos + 1);
}
row.push_back(line); // 添加最后一个元素
data.push_back(row);
}
// 打印读取到的数据
for (const auto& row : data) {
for (const auto& cell : row) {
std::cout << cell << "\t";
}
std::cout << std::endl;
}
} else {
std::cout << "无法打开文件" << std::endl;
}
file.close();
return 0;
}
```
在上面的示例代码中,我们打开名为 "data.csv" 的CSV文件,并使用std::ifstream来读取文件内容。然后,我们逐行读取文件,并将每一行按逗号分割成不同的单元格。最后,我们将每一行的数据存储在一个二维vector中,并打印出来。
请确保将文件名 "data.csv" 替换为你实际使用的CSV文件的路径和文件名。此外,还要注意确保文件存在并可读取。
阅读全文
相关推荐
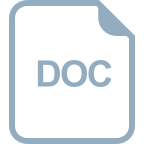
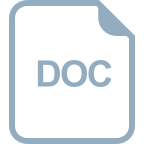
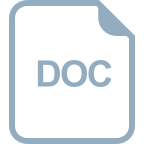
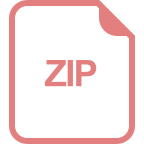

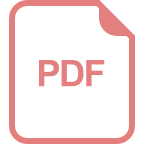
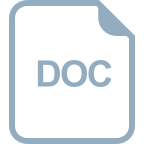
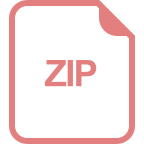









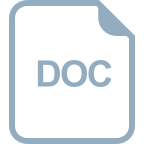