c++类与对象,作book,student,phone三个类并进行功能的编程
时间: 2024-09-22 21:08:37 浏览: 35
在C++中,类是一种用户自定义的数据类型,它封装数据(属性)和操作数据的方式(函数或成员函数)。让我们创建`Book`, `Student`, 和 `Phone` 这三个类,并赋予它们一些基本的功能。
```cpp
// Book 类
class Book {
public:
// 构造函数
Book(string title, int pages) : title(title), pages(pages) {}
// 属性
string getTitle() const { return title; }
int getPages() const { return pages; }
// 操作函数 - 显示书的信息
void displayInfo() {
cout << "Title: " << title << ", Pages: " << pages << endl;
}
private:
string title;
int pages;
};
// Student 类继承自 Book 并添加学生特有属性
class Student : public Book {
public:
Student(string name, int id, string bookTitle, int bookPages)
: Book(bookTitle, bookPages), name(name), id(id) {}
// 学生特有的属性和操作
string getName() const { return name; }
int getId() const { return id; }
void introduce() {
cout << "Name: " << name << ", ID: " << id << ", Reading: " << getTitle() << endl;
}
private:
string name;
int id;
};
// Phone 类
class Phone {
public:
Phone(string model, string brand) : model(model), brand(brand) {}
// 属性
string getModel() const { return model; }
string getBrand() const { return brand; }
// 操作函数 - 显示电话信息
void displayInfo() {
cout << "Model: " << model << ", Brand: " << brand << endl;
}
private:
string model;
string brand;
};
```
现在你可以实例化这些类并调用其方法:
```cpp
int main() {
// 创建一个Book对象
Book myBook("Programming Basics", 400);
myBook.displayInfo();
// 创建一个Student对象,继承自Book
Student myStudent("Alice", 123456, "Algorithms", 700);
myStudent.introduce();
// 创建一个Phone对象
Phone myPhone("iPhone 13", "Apple");
myPhone.displayInfo();
return 0;
}
```
阅读全文
相关推荐
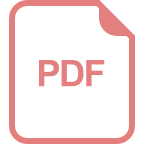
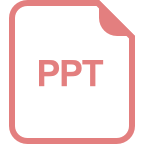
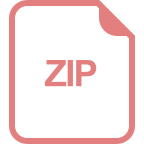
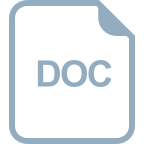
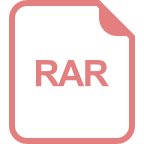
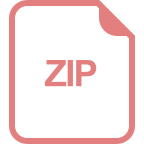
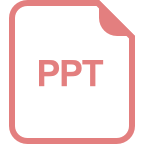
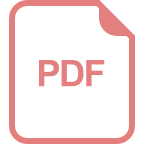
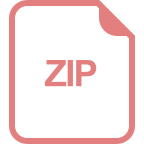
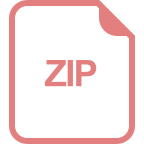
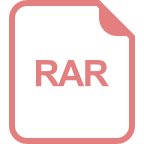
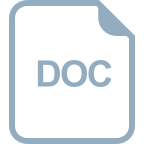
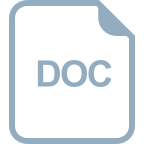
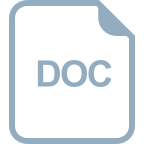
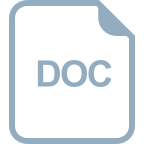
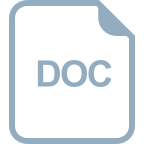
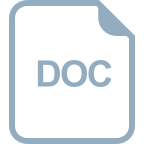
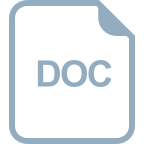