运行时有错误,怎么改代码才能得到结果?
时间: 2024-12-20 12:30:12 浏览: 8
根据您提供的代码内容,存在一些潜在的问题可能导致运行错误。以下是可能的改进建议:
### 1. 导入包检查
确保所有的包都已经正确导入,特别是 `scipy` 和 `tqdm`。如果未安装这些包,请使用以下命令进行安装:
```bash
pip install scipy tqdm
```
### 2. 函数参数和返回值的一致性
在 `calculate_distances` 函数中,传递给 `pdist` 的 `points` 列表应仅包含经纬度坐标。当前的实现可能会导致形状不匹配。修改如下:
```python
def calculate_distances(sources, centers, landfills, manufacturing_centers):
points = []
for _, lat, lon, _ in sources:
points.append((lat, lon))
for _, lat, lon, _, _, _, _ in centers:
points.append((lat, lon))
for _, lat, lon, _, _, _, _ in manufacturing_centers:
points.append((lat, lon))
for _, lat, lon, _, _ in landfills:
points.append((lat, lon))
dist = pdist(points, metric=lambda u, v: haversine(u[0], u[1], v[0], v[1]))
return squareform(dist)
```
### 3. 粒子初始化和更新逻辑
粒子的位置应该在合理的范围内。例如,对于节点选择,粒子的位置应该是从 0 开始到对应节点数量减 1 的整数。可以通过 `numpy` 的 `randint` 方法来生成合适的初始位置:
```python
def initialize_population(num_particles, num_dimensions, lower_bound, upper_bound):
particles = np.random.randint(lower_bound, upper_bound, size=(num_particles, num_dimensions))
velocities = np.zeros_like(particles)
personal_best_positions = particles.copy()
personal_best_values = np.full(num_particles, float('inf'))
global_best_position = None
global_best_value = float('inf')
return particles, velocities, personal_best_positions, personal_best_values, global_best_position, global_best_value
```
### 4. 粒子更新逻辑
在更新粒子位置时,需要确保新位置仍然在合理范围内:
```python
def update_particles(particles, velocities, personal_best_positions, global_best_position, w=0.5, c1=2, c2=2, lower_bound=0, upper_bound=None):
r1 = np.random.rand(*particles.shape)
r2 = np.random.rand(*particles.shape)
velocities = w * velocities + c1 * r1 * (personal_best_positions - particles) + c2 * r2 * (global_best_position - particles)
new_positions = particles + velocities
# 确保新的位置仍在合理范围内
new_positions = np.clip(new_positions, lower_bound, upper_bound)
return new_positions, velocities
```
### 5. 主程序部分
确保在主程序中传入正确的下界和上界:
```python
if __name__ == "__main__":
distances = calculate_distances(waste_sources, recycling_centers, landfills, remanufacturing_centers)
num_dimensions = len(waste_sources) + len(recycling_centers) + len(remanufacturing_centers) + len(landfills)
lower_bound = 0
upper_bound = len(waste_sources) + len(recycling_centers) + len(remanufacturing_centers) + len(landfills) - 1
best_costs, best_emissions, global_best_position = pso_main(
distances,
waste_sources,
recycling_centers,
remanufacturing_centers,
landfills,
max_iterations=100,
num_particles=30,
verbose=True,
lower_bound=lower_bound,
upper_bound=upper_bound
)
# 输出总成本和总碳排放量
print(f"Final Total Cost: {best_costs[-1]}")
print(f"Final Total Emission: {best_emissions[-1]}")
# 提取并输出选中的节点
selected_recycling_nodes = [int(pos) for pos in global_best_position[len(waste_sources): len(waste_sources) + len(recycling_centers)]]
selected_remanufacturing_nodes = [int(pos) for pos in global_best_position[len(waste_sources) + len(recycling_centers):-len(landfills)]]
print("Selected Recycling Nodes:")
for i, node in enumerate(selected_recycling_nodes):
print(f"Recycling Center {i + 1}: Node {node}")
print("Selected Remanufacturing Nodes:")
for i, node in enumerate(selected_remanufacturing_nodes):
print(f"Remanufacturing Center {i + 1}: Node {node}")
# 绘制收敛曲线
fig, ax1 = plt.subplots()
ax1.plot(best_costs, 'r-', label='Cost')
ax1.set_xlabel('Iterations')
ax1.set_ylabel('Total Cost')
ax2 = ax1.twinx()
ax2.plot(best_emissions, 'b-', label='Emission')
ax2.set_ylabel('Total Emission')
plt.title('Convergence of PSO Algorithm')
fig.tight_layout()
plt.show()
```
通过以上改进,您的代码应该能够正常运行并得到预期的结果。如果仍有问题,请提供具体的错误信息以便进一步调试。
阅读全文
相关推荐
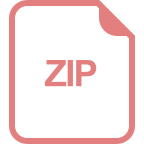
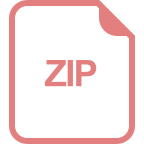
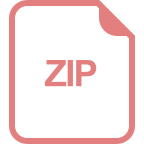

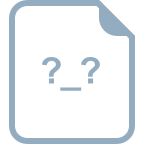
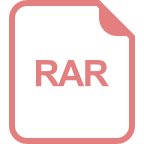
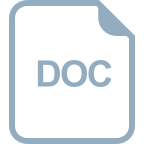
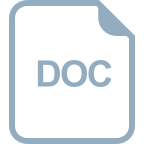
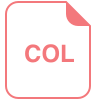
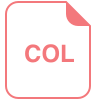
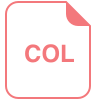
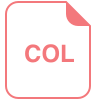
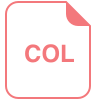
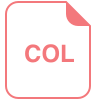
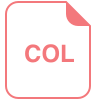
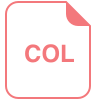
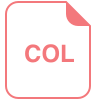
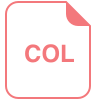
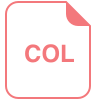