面向对象程序设计java汽车租赁
时间: 2023-11-20 12:56:29 浏览: 195
面向对象程序设计Java汽车租赁是一种基于Java语言和MySQL数据库的汽车租赁管理系统。该系统可以实现汽车的租赁、归还、查询、统计等功能。以下是该系统的主要功能模块:
1.用户管理模块:实现用户的注册、登录、修改密码等功能。
2.汽车管理模块:实现汽车的添加、删除、修改、查询等功能。
3.租赁管理模块:实现租赁订单的生成、查询、修改、删除等功能。
4.归还管理模块:实现归还订单的生成、查询、修改、删除等功能。
5.统计管理模块:实现租赁订单和归还订单的统计功能。
以下是一个Java汽车租赁管理系统的示例代码:
```java
//汽车类
public class Car {
private String carId; //汽车编号
private String carName; //汽车名称
private double price; //租赁价格
private boolean isRent; //是否已被租赁
//构造方法
public Car(String carId, String carName, double price) {
this.carId = carId;
this.carName = carName;
this.price = price;
this.isRent = false;
}
//getter和setter方法
public String getCarId() {
return carId;
}
public void setCarId(String carId) {
this.carId = carId;
}
public String getCarName() {
return carName;
}
public void setCarName(String carName) {
this.carName = carName;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public boolean isRent() {
return isRent;
}
public void setRent(boolean rent) {
isRent = rent;
}
}
//租赁订单类
public class RentOrder {
private String orderId; //订单编号
private String userId; //用户编号
private String carId; //汽车编号
private Date rentDate; //租赁日期
private Date returnDate; //归还日期
private double rentPrice; //租赁价格
//构造方法
public RentOrder(String orderId, String userId, String carId, Date rentDate, Date returnDate, double rentPrice) {
this.orderId = orderId;
this.userId = userId; this.carId = carId;
this.rentDate = rentDate;
this.returnDate = returnDate;
this.rentPrice = rentPrice;
}
//getter和setter方法
public String getOrderId() {
return orderId;
}
public void setOrderId(String orderId) {
this.orderId = orderId;
}
public String getUserId() {
return userId;
}
public void setUserId(String userId) {
this.userId = userId;
}
public String getCarId() {
return carId;
}
public void setCarId(String carId) {
this.carId = carId;
}
public Date getRentDate() {
return rentDate;
}
public void setRentDate(Date rentDate) {
this.rentDate = rentDate;
}
public Date getReturnDate() {
return returnDate;
}
public void setReturnDate(Date returnDate) {
this.returnDate = returnDate;
}
public double getRentPrice() {
return rentPrice;
}
public void setRentPrice(double rentPrice) {
this.rentPrice = rentPrice;
}
}
//租赁系统类
public class RentCarSys {
private List<Car> carList; //汽车列表
private List<RentOrder> rentOrderList; //租赁订单列表
private Scanner scanner; //输入流
//构造方法
public RentCarSys() {
this.carList = new ArrayList<>();
this.rentOrderList = new ArrayList<>();
this.scanner = new Scanner(System.in);
}
//添加汽车
public void addCar() {
System.out.println("请输入汽车编号:");
String carId = scanner.nextLine();
System.out.println("请输入汽车名称:");
String carName = scanner.nextLine();
System.out.println("请输入租赁价格:");
double price = scanner.nextDouble();
scanner.nextLine();
Car car = new Car(carId, carName, price);
carList.add(car);
System.out.println("添加成功!");
}
//删除汽车
public void deleteCar() {
System.out.println("请输入汽车编号:");
String carId = scanner.nextLine();
for (Car car : carList) {
if (car.getCarId().equals(carId)) {
carList.remove(car);
System.out.println("删除成功!");
return;
}
}
System.out.println("未找到该汽车!");
}
//修改汽车信息
public void modifyCar() {
System.out.println("请输入汽车编号:");
String carId = scanner.nextLine();
for (Car car : carList) {
if (car.getCarId().equals(carId)) {
System.out.println("请输入新的汽车名称:");
String carName = scanner.nextLine();
System.out.println("请输入新的租赁价格:");
double price = scanner.nextDouble();
scanner.nextLine();
car.setCarName(carName);
car.setPrice(price);
System.out.println("修改成功!");
return;
}
}
System.out.println("未找到该汽车!");
}
//查询汽车信息
public void queryCar() {
System.out.println("请输入汽车编号:");
String carId = scanner.nextLine();
for (Car car : carList) {
if (car.getCarId().equals(carId)) {
System.out.println("汽车编号:" + car.getCarId());
System.out.println("汽车名称:" + car.getCarName());
System.out.println("租赁价格:" + car.getPrice());
System.out.println("是否已被租赁:" + car.isRent());
return;
}
}
System.out.println("未找到该汽车!");
}
//生成租赁订单
public void generateRentOrder() {
System.out.println("请输入用户编号:");
String userId = scanner.nextLine();
System.out.println("请输入汽车编号:");
String carId = scanner.nextLine();
for (Car car : carList) {
if (car.getCarId().equals(carId)) {
if (car.isRent()) {
System.out.println("该汽车已被租赁!");
return;
} else {
System.out.println("请输入租赁日期(格式为yyyy-MM-dd):");
String rentDateStr = scanner.nextLine();
Date rentDate = null;
try {
rentDate = new SimpleDateFormat("yyyy-MM-dd").parse(rentDateStr);
} catch (ParseException e) {
System.out.println("日期格式错误!");
return;
}
System.out.println("请输入归还日期(格式为yyyy-MM-dd):");
String returnDateStr = scanner.nextLine();
Date returnDate = null;
try {
returnDate = new SimpleDateFormat("yyyy-MM-dd").parse(returnDateStr);
} catch (ParseException e) {
System.out.println("日期格式错误!");
return;
}
double rentPrice = (returnDate.getTime() - rentDate.getTime()) / (1000 * 60 * 60 * 24) * car.getPrice();
RentOrder rentOrder = new RentOrder(UUID.randomUUID().toString(), userId, carId, rentDate, returnDate, rentPrice);
rentOrderList.add(rentOrder);
car.setRent(true);
System.out.println("租赁成功!");
return;
}
}
}
System.out.println("未找到该汽车!");
}
//归还租赁订单
public void returnRentOrder() {
System.out.println("请输入订单编号:");
String orderId = scanner.nextLine();
for (RentOrder rentOrder : rentOrderList) {
if (rentOrder.getOrderId().equals(orderId)) {
rentOrderList.remove(rentOrder);
for (Car car : carList) {
if (car.getCarId().equals(rentOrder.getCarId())) {
car.setRent(false);
break;
}
}
System.out.println("归还成功!");
return;
}
}
System.out.println("未找到该订单!");
}
//统计租赁订单和归还订单
public void countOrder() {
System.out.println("请输入统计类型(1-租赁订单,2-归还订单):");
int type = scanner.nextInt();
scanner.nextLine();
System.out.println("请输入统计开始日期(格式为yyyy-MM-dd):");
String startDateStr = scanner.nextLine();
Date startDate = null;
try {
startDate = new SimpleDateFormat("yyyy-MM-dd").parse(startDateStr);
} catch (ParseException e) {
System.out.println("日期格式错误!");
return;
}
System.out.println("请输入统计结束日期(格式为yyyy-MM-dd):");
String endDateStr = scanner.nextLine();
Date endDate = null;
try {
endDate = new SimpleDateFormat("yyyy-MM-dd").parse(endDateStr);
} catch (ParseException e) {
System.out.println("日期格式错误!");
return;
}
int count = 0;
double totalPrice = 0;
if (type == 1) {
for (RentOrder rentOrder : rentOrderList) {
if (rentOrder.getRentDate().getTime() >= startDate.getTime() && rentOrder.getRentDate().getTime() <= endDate.getTime()) {
count++;
totalPrice += rentOrder.getRentPrice();
}
}
System.out.println("租赁订单数量:" + count);
System.out.println("租赁订单总金额:" + totalPrice);
} else if (type == 2) {
for (RentOrder rentOrder : rentOrderList) {
if (rentOrder.getReturnDate().getTime() >= startDate.getTime() && rentOrder.getReturnDate().getTime() <= endDate.getTime()) {
count++;
totalPrice += rentOrder.getRentPrice();
}
}
System.out.println("归还订单数量:" + count);
System.out.println("归还订单总金额:" + totalPrice);
} else {
System.out.println("统计类型错误!");
}
}
//启动租赁系统
public void starRentCarSys() throws IOException, OverChoiceException, ErrorSetException {
System.out.println("欢迎使用汽车租赁管理系统!");
while (true) {
System.out
阅读全文
相关推荐

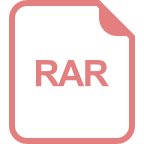
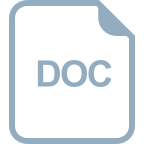
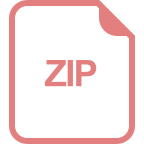
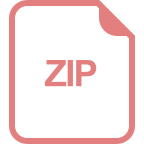
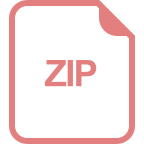
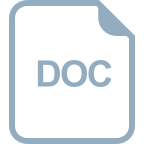
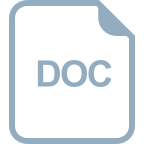
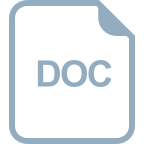
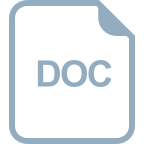
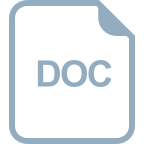
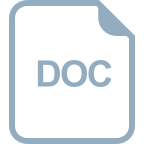
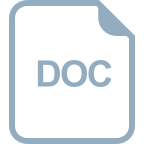
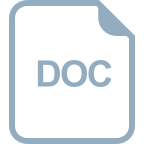
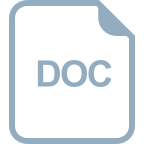
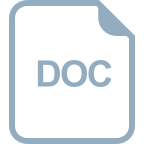