unity帮我写一下种田系统和天气系统
时间: 2024-10-03 15:03:27 浏览: 23
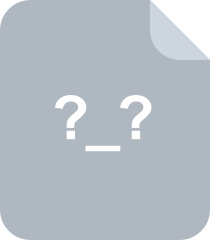
Unity天气系统(Enviro - Sky and Weather)插件

在Unity中创建一个种田系统和天气系统涉及到游戏逻辑、场景设置以及可能的数据管理。这里我会给你提供一些基本的思路和代码片段,但请注意这只是一个基础框架,实际开发中你需要根据项目需求进行详细的定制。
**种田系统(Planting System)**
1. **创建资源**:
- 设计种子对象(比如种子Prefab),包含生长速度、收获时间等属性。
- 创建田地(Farmland)或地块(GridCell)组件,用于存储种植状态和当前作物。
```csharp
public class Plant : MonoBehaviour
{
public int growthSpeed;
public float harvestTime;
// ...
}
```
2. **播种逻辑**:
- 当玩家选择种植,检查田地是否有空位,然后放置种子并更新其生长状态。
```csharp
public void PlantSeed(PlantType type, Vector3 position)
{
if (Farmland.CanPlant(position))
{
Farmland.Instance.PlacePlant(position, type);
}
}
```
3. **收获逻辑**:
- 定期检查作物是否成熟,成熟后移除植物并增加收益。
```csharp
void Update()
{
foreach (Plant plant in Farmland.Instance.GetPlants())
{
if (plant.IsHarvestable())
{
// 收获并更新田地
RemovePlantAt(plant.transform.position);
}
}
}
```
**天气系统(Weather System)**:
1. **添加天气组件**:
- 创建一个WeatherManager类负责控制天气变化。
```csharp
public class WeatherManager : MonoBehaviour
{
public List<Weather> weatherStates;
private Weather currentWeather;
// ...
}
```
2. **天气状态管理**:
- 用枚举或字典定义不同的天气状态(晴天、雨天、风暴等),每个状态都有对应的效果和持续时间。
```csharp
public enum WeatherState
{
Sunny,
Rainy,
Stormy
}
private void ChangeWeather(WeatherState state)
{
currentWeather?.FadeOut();
currentWeather = weatherStates[state];
currentWeather.FadeIn();
}
```
3. **随机或定时切换天气**:
- 可以用`Random.Range`生成随机数决定天气变化,或者设定时间间隔轮换天气。
```csharp
void UpdateWeather()
{
// 随机选择新天气
RandomIndex = Random.Range(0, weatherStates.Count);
// 检查是否到切换时间
if (currentWeather.ChangeTime <= Time.time)
{
ChangeWeather(weatherStates[RandomIndex]);
}
}
```
阅读全文
相关推荐
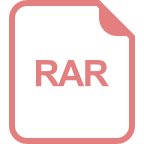
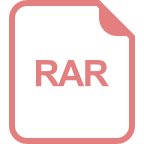















