语言如何将输入的字符串的ASCII码加3转化为另一个字符串后输出
时间: 2024-10-19 09:04:22 浏览: 22
在计算机编程中,如果你想把输入的字符串每个字符的ASCII码值增加3,并转换成新的字符串输出,你可以按照以下步骤操作:
1. 遍历输入字符串的每一个字符。
2. 对于每个字符,获取其对应的ASCII码值(在Python中可以使用ord()函数)。
3. 将ASCII码值加上3。
4. 将处理后的ASCII码值转换回字符(在Python中可以使用chr()函数)。
5. 将新字符添加到一个新的字符串中。
6. 遍历完成后,返回这个包含所有字符ASCII码加3的新字符串。
下面是一个简单的Python示例:
```python
def ascii_add_three(input_string):
new_string = ""
for char in input_string:
new_code = ord(char) + 3
new_char = chr(new_code)
new_string += new_char
return new_string
# 测试例子
input_str = "Hello"
output_str = ascii_add_three(input_str)
print(f"原始字符串: {input_str}")
print(f"ASCII加3后的字符串: {output_str}")
```
运行此程序,会得到每个字符ASCII码值加3的结果。
相关问题
c语言如何将输入的字符串的ASCII码加3转化为另一个字符串后输出
在C语言中,你可以通过以下步骤将输入的字符串每个字符的ASCII值加3,然后转换回对应的字符并输出到新的字符串:
1. **定义函数**:首先,你需要创建一个函数,如`ascii_plus_three()`,该函数接收一个指向输入字符串的指针,并返回一个新的字符串。
```c
#include <stdio.h>
#include <string.h>
char* ascii_plus_three(const char* input) {
int len = strlen(input);
char output[len + 1]; // 预留空间用于新字符串
for (int i = 0; i < len; ++i) {
// 将输入字符的ASCII值加3,处理超出范围的情况
char new_char = ((input[i] - 'a' + 3) % 26) + 'a'; // 对于小写字母
if (new_char > 'z') { // 大写字母的话加上26(ASCII z 到 ASCII A)
new_char = (new_char - 'a' + 26) % 26 + 'A';
}
output[i] = new_char;
}
output[len] = '\0'; // 结束符
return output;
}
```
2. **主程序调用**:在主函数main()中,获取用户输入的字符串,然后调用`ascii_plus_three()`函数,并打印结果。
```c
int main() {
char input[100];
printf("请输入一个字符串: ");
fgets(input, sizeof(input), stdin); // 注意去除行结束符'\n'
input[strcspn(input, "\n")] = '\0'; // 去除fgets读取的换行符
char* result = ascii_plus_three(input);
printf("转换后的字符串: %s\n", result);
free(result); // 如果需要,释放内存
return 0;
}
```
C语言循环语句如何将输入的字符串的ASCII码加3转化为另一个字符串后输出
在C语言中,你可以通过遍历输入字符串并逐个字符地对ASCII值加3来实现这个转换。这里是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
void increment_ascii(char *input_string) {
int len = strlen(input_string);
for (int i = 0; i < len; i++) {
char original_char = input_string[i];
if (original_char >= 'a' && original_char <= 'z') { // 对小写字母操作
char incremented_char = original_char + 3 - 'a'; // 如果是a-z,直接加3,否则会溢出
if (incremented_char > 'z') { // 避免超出z
incremented_char -= 26;
}
input_string[i] = incremented_char;
} else if (original_char >= 'A' && original_char <= 'Z') { // 对大写字母操作
char incremented_char = original_char + 3 - 'A';
if (incremented_char > 'Z') {
incremented_char -= 26;
}
input_string[i] = incremented_char;
} else { // 对其他非字母字符不做处理
continue;
}
}
}
// 输出转换后的字符串
void print_string(char *output_string) {
printf("%s", output_string);
}
int main() {
char input[100];
printf("Enter a string: ");
fgets(input, sizeof(input), stdin); // 读取一行输入,包括换行符
input[strlen(input) - 1] = '\0'; // 去除换行符
char output[100];
increment_ascii(input);
output_string = input; // 将修改后的字符串赋值给输出变量
print_string(output);
return 0;
}
```
阅读全文
相关推荐
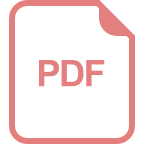
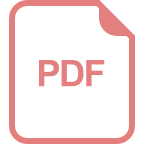


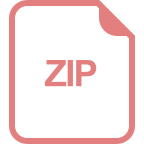








