如何用QPixmap实例化QIcon
时间: 2024-06-13 07:08:06 浏览: 230
可以使用QIcon的fromTheme()方法或者QIcon的QPixmap()方法来实例化QIcon。其中,QPixmap()方法需要传入一个QPixmap对象作为参数。下面是使用QPixmap()方法实例化QIcon的示例代码:
```python
from PyQt5.QtGui import QIcon, QPixmap
from PyQt5.QtWidgets import QApplication, QLabel
app = QApplication([])
label = QLabel()
# 实例化QPixmap对象
pixmap = QPixmap('E:\pyqt5快速开发与实战\第四章\images\python.png')
# 使用QPixmap()方法实例化QIcon
icon = QIcon(pixmap)
# 将QIcon设置为QLabel的图标
label.setPixmap(pixmap)
# 显示QLabel
label.show()
app.exec_()
```
相关问题
#include "mylogin.h" mylogin::mylogin(QWidget *parent) : QDialog(parent) { this->init_ui(); connect(this->bnt_login, &QPushButton::clicked, this, &mylogin::do_login); connect(this->bnt_register, &QPushButton::clicked , this ,&mylogin::do_enroll); } mylogin::~mylogin() { } void mylogin::init_ui() { this->setFixedSize(QSize(600,350)); this->setWindowTitle(tr("岑超升")); this->setWindowIcon(QIcon(":/src/1.png")); this->lb1 = new QLabel(); this->lb2 = new QLabel(); this->lb3 = new QLabel(); this->lb1->setFixedSize(QSize(560,200)); QPixmap pic; pic.load(":/src/2.png"); //this->lb1->setPixmap(pic.scaled(this->lb1->size(), Qt::KeepAspectRatio, Qt::SmoothTransformation)); this->lb1->setPixmap(QPixmap(":/src/2.png")); this->lb2->setText(tr("用户名:")); this->lb3->setText(tr("密 码:")); this->usr_name_le = new QLineEdit(); //这两个参数后面传入信号中去,然后emit发射出去 this->usr_pass_le = new QLineEdit(); this->usr_pass_le->setEchoMode(QLineEdit::Password); this->bnt_login = new QPushButton(tr("登 陆")); this->bnt_register = new QPushButton(tr("注 册")); this->hb1 = new QHBoxLayout(); this->hb2 = new QHBoxLayout(); this->hb3 = new QHBoxLayout(); this->hb1->addWidget(this->lb2); this->hb1->addWidget(this->usr_name_le); this->hb2->addWidget(this->lb3); this->hb2->addWidget(this->usr_pass_le); this->hb3->addWidget(this->bnt_login); this->hb3->addWidget(this->bnt_register); this->vb1 = new QVBoxLayout(); this->vb1->addWidget(this->lb1); this->vb1->addLayout(this->hb1); this->vb1->addLayout(this->hb2); this->vb1->addLayout(this->hb3); this->setLayout(this->vb1); } void mylogin::do_login() { emit sig_login(usr_name_le->text(), usr_pass_le->text()); //需要把这里输入的账号密码信息发送到myapp那去,用到的函数是emit //需要自定义一个信号,sig_login //这个槽函数能够发出信号 } void mylogin::do_enroll() { emit sig_enroll(); }用C++语言解释并注释每行代码,并说明他们的功能和作用
```c++
#include "mylogin.h" //引入自定义头文件
mylogin::mylogin(QWidget *parent) : QDialog(parent) { //构造函数,继承自QDialog类
this->init_ui(); //调用init_ui函数
connect(this->bnt_login, &QPushButton::clicked, this, &mylogin::do_login); //连接信号和槽函数
connect(this->bnt_register, &QPushButton::clicked , this ,&mylogin::do_enroll); //连接信号和槽函数
}
mylogin::~mylogin() { //析构函数
}
void mylogin::init_ui() { //初始化UI的函数
this->setFixedSize(QSize(600,350)); //设置窗口大小
this->setWindowTitle(tr("岑超升")); //设置窗口标题
this->setWindowIcon(QIcon(":/src/1.png")); //设置窗口图标
this->lb1 = new QLabel(); //实例化QLabel对象
this->lb2 = new QLabel(); //实例化QLabel对象
this->lb3 = new QLabel(); //实例化QLabel对象
this->lb1->setFixedSize(QSize(560,200)); //设置QLabel的大小
QPixmap pic; //实例化QPixmap对象
pic.load(":/src/2.png"); //加载图片
this->lb1->setPixmap(QPixmap(":/src/2.png")); //设置QLabel中显示的图片
this->lb2->setText(tr("用户名:")); //设置QLabel中显示的文本
this->lb3->setText(tr("密 码:")); //设置QLabel中显示的文本
this->usr_name_le = new QLineEdit(); //实例化QLineEdit对象
this->usr_pass_le = new QLineEdit(); //实例化QLineEdit对象
this->usr_pass_le->setEchoMode(QLineEdit::Password); //设置QLineEdit中输入的内容显示方式
this->bnt_login = new QPushButton(tr("登 陆")); //实例化QPushButton对象
this->bnt_register = new QPushButton(tr("注 册")); //实例化QPushButton对象
this->hb1 = new QHBoxLayout(); //实例化QHBoxLayout对象
this->hb2 = new QHBoxLayout(); //实例化QHBoxLayout对象
this->hb3 = new QHBoxLayout(); //实例化QHBoxLayout对象
this->hb1->addWidget(this->lb2); //将QLabel添加到QHBoxLayout中
this->hb1->addWidget(this->usr_name_le); //将QLineEdit添加到QHBoxLayout中
this->hb2->addWidget(this->lb3); //将QLabel添加到QHBoxLayout中
this->hb2->addWidget(this->usr_pass_le); //将QLineEdit添加到QHBoxLayout中
this->hb3->addWidget(this->bnt_login); //将QPushButton添加到QHBoxLayout中
this->hb3->addWidget(this->bnt_register); //将QPushButton添加到QHBoxLayout中
this->vb1 = new QVBoxLayout(); //实例化QVBoxLayout对象
this->vb1->addWidget(this->lb1); //将QLabel添加到QVBoxLayout中
this->vb1->addLayout(this->hb1); //将QHBoxLayout添加到QVBoxLayout中
this->vb1->addLayout(this->hb2); //将QHBoxLayout添加到QVBoxLayout中
this->vb1->addLayout(this->hb3); //将QHBoxLayout添加到QVBoxLayout中
this->setLayout(this->vb1); //设置布局
}
void mylogin::do_login() { //登录槽函数
emit sig_login(usr_name_le->text(), usr_pass_le->text()); //发射信号
}
void mylogin::do_enroll() { //注册槽函数
emit sig_enroll(); //发射信号
}
```
以上代码为一个简单的登录界面的实现,主要是通过Qt中的QLabel、QLineEdit、QPushButton、QHBoxLayout、QVBoxLayout等控件和布局来实现。其中,`init_ui`函数用于初始化UI界面,`do_login`和`do_enroll`函数分别为登录和注册的槽函数,并且通过`connect`函数将信号和槽函数连接起来,实现登录和注册的功能。
Got keys from plugin meta data ("ibus") QFactoryLoader::QFactoryLoader() checking directory path "/usr/bin/platforminputcontexts" ... loaded library "/home/rvbust/.local/lib/python3.10/site-packages/PyQt5/Qt5/plugins/platforminputcontexts/libcomposeplatforminputcontextplugin.so" QFactoryLoader::QFactoryLoader() checking directory path "/home/rvbust/.local/lib/python3.10/site-packages/PyQt5/Qt5/plugins/styles" ... QFactoryLoader::QFactoryLoader() checking directory path "/usr/bin/styles" ... Traceback (most recent call last): File "/home/rvbust/Documents/FlexLocation_new/ViewerApp.py", line 30, in <module> main() File "/home/rvbust/Documents/FlexLocation_new/ViewerApp.py", line 24, in main main_window = GlyphViewerApp() File "/home/rvbust/Documents/FlexLocation_new/ViewerApp.py", line 10, in __init__ self.setup() File "/home/rvbust/Documents/FlexLocation_new/ViewerApp.py", line 15, in setup self.ui.setupUi(self) File "/home/rvbust/Documents/FlexLocation_new/glyph_view.py", line 33, in setupUi self.actionLoadCloud = QAction(MainWindow) TypeError: 'PySide6.QtGui.QAction.__init__' called with wrong argument types: PySide6.QtGui.QAction.__init__(GlyphViewerApp) Supported signatures: PySide6.QtGui.QAction.__init__(Union[PySide6.QtGui.QIcon, PySide6.QtGui.QPixmap], str, Optional[PySide6.QtCore.QObject] = None) PySide6.QtGui.QAction.__init__(Optional[PySide6.QtCore.QObject] = None) PySide6.QtGui.QAction.__init__(str, Optional[PySide6.QtCore.QObject] = None) QLibraryPrivate::unload succeeded on "/home/rvbust/.local/lib/python3.10/site-packages/PyQt5/Qt5/plugins/platforminputcontexts/libcomposeplatforminputcontextplugin.so" QLibraryPrivate::unload succeeded on "/home/rvbust/.local/lib/python3.10/site-packages/PyQt5/Qt5/plugins/platformthemes/libqgtk3.so" QLibraryPrivate::unload succeeded on "/home/rvbust/.local/lib/python3.10/site-packages/PyQt5/Qt5/plugins/platforms/libqxcb.so" QLibraryPrivate::unload succeeded on "Xcursor" (faked)
这个错误信息出现在实例化 `GlyphViewerApp` 对象时,调用了 `setup` 方法。具体来说,`GlyphViewerApp` 继承自 `QtWidgets.QMainWindow`,而 `setup` 方法中又调用了 `glyph_view.ui` 模块中的 `setupUi` 方法创建了一些 UI 控件,其中包括一个 `QAction` 对象。但是在创建 `QAction` 对象时,传入的参数类型不正确,导致了这个错误。
解决这个问题的方法是,在 `glyph_view.ui` 模块中找到创建 `QAction` 对象的代码,确保参数类型正确。根据错误信息中的提示,`QAction.__init__` 方法支持多种不同的参数类型,需要根据实际情况选择正确的参数类型传入。例如,可以尝试修改为:
```python
self.actionLoadCloud = QAction("Load Cloud", self)
```
这里将第一个参数修改为字符串类型,表示 `QAction` 对象的文本标签。同时,将第二个参数指定为 `self`,表示 `QAction` 对象的父对象为 `GlyphViewerApp` 对象。这样就可以避免参数类型不匹配的错误了。
阅读全文
相关推荐
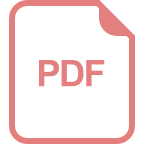
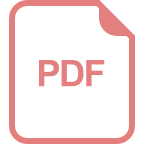
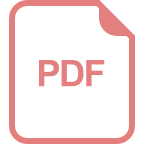
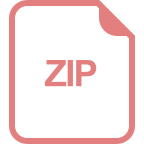
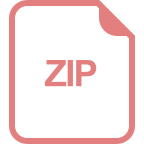
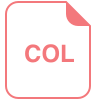

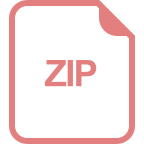
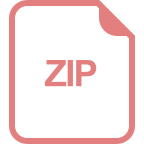
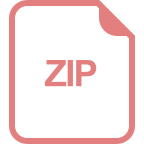
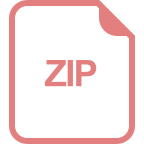