用c++写一个可视化迷宫游戏,并给出代码
时间: 2023-08-31 08:35:02 浏览: 264
### 回答1:
嗯,我可以用C语言来写一个可视化迷宫游戏,代码如下:#include<stdio.h>
#include<conio.h>
#define MAX 100//定义迷宫的边界
int x_min=2;
int x_max=MAX-3;
int y_min=2;
int y_max=MAX-3;//定义游戏的初始状态
int x=2;
int y=2;
int flag=0;char map[MAX][MAX]={"#####","# #","# #","# #","# #","# #","# #","#####"}; //游戏的主函数
int main()
{
int i,j;
//初始化游戏界面
printf("欢迎来到迷宫游戏!\n");
while(1)
{
//输出游戏界面
for(i=0;i<MAX;i++)
{
for(j=0;j<MAX;j++)
{
if(i==x&&j==y)
printf("@");
else
printf("%c",map[i][j]);
}
printf("\n");
}
//接受按键指令
char key;
key=getch();
switch(key)
{
case 'w':
{
if(x-1>x_min)
x--;
break;
}
case 's':
{
if(x+1<x_max)
x++;
break;
}
case 'a':
{
if(y-1>y_min)
y--;
break;
}
case 'd':
{
if(y+1<y_max)
y++;
break;
}
case 'q':
{
printf("退出游戏!\n");
return 0;
}
}
//判断游戏是否结束
if(x==x_max&&y==y_max)
{
printf("恭喜你,成功逃出迷宫!\n");
return 0;
}
}
return 0;
}
### 回答2:
以下是使用C语言编写的简单可视化迷宫游戏的代码:
```c
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
#include <windows.h>
#define WIDTH 10
#define HEIGHT 10
char maze[HEIGHT][WIDTH] = {
{'#', '#', '#', '#', '#', '#', '#', '#', '#', '#'},
{'#', '.', '.', '.', '#', '.', '.', '.', '.', '#'},
{'#', '.', '#', '.', '#', '.', '#', '#', '.', '#'},
{'#', '.', '#', '.', 'O', 'O', '.', '#', '.', '#'},
{'#', '.', '#', '#', '#', '.', '#', '#', '.', '#'},
{'#', '.', '#', '.', '#', '.', '.', '.', '.', '#'},
{'#', '.', '.', '.', '#', '#', '#', '#', '.', '#'},
{'#', '#', '#', '#', '#', '#', '#', '#', '#', '#'},
};
void drawMaze() {
int i, j;
for (i = 0; i < HEIGHT; i++) {
for (j = 0; j < WIDTH; j++) {
printf("%c", maze[i][j]);
}
printf("\n");
}
}
int main() {
int posX = 1;
int posY = 1;
char input;
maze[posY][posX] = 'X';
while (1) {
system("cls");
drawMaze();
if (posX == 8 && posY == 3) {
printf("\nCongratulations, you solved the maze!\n");
break;
}
input = getch();
maze[posY][posX] = '.';
if (input == 'w' && maze[posY - 1][posX] != '#') posY--;
else if (input == 's' && maze[posY + 1][posX] != '#') posY++;
else if (input == 'a' && maze[posY][posX - 1] != '#') posX--;
else if (input == 'd' && maze[posY][posX + 1] != '#') posX++;
maze[posY][posX] = 'X';
}
return 0;
}
```
以上代码使用字符数组来表示迷宫地图,其中'#'表示墙壁,'.'表示可通过的路径,'O'表示迷宫的终点,'X'表示当前位置。玩家通过键盘输入w、a、s、d来控制移动方向,直到找到终点位置时游戏结束并显示相应的提示信息。
### 回答3:
这里是一个简单的使用C语言编写的可视化迷宫游戏的代码示例:
```c
#include <stdio.h>
#include <conio.h>
#define WIDTH 10
#define HEIGHT 10
void drawMaze(int playerX, int playerY) {
char maze[HEIGHT][WIDTH] = {
"+-+-+-+-+-+-+-+-+-+",
"| |",
"+-+ +-+ +-+ +-+ +-+",
"| | | | |",
"+-+ + +-+-+-+ +-+ +",
"| | | |",
"+-+-+-+-+-+-+ + +-+",
"| | | |",
"+ +-+ +-+ + +-+ + +-+",
"| | | | | |",
"+-+ +-+ +-+-+-+-+-+",
"| | |",
"+-+-+-+-+-+-+-+-+-+"
};
maze[playerY][playerX] = 'P';
system("cls");
for (int y = 0; y < HEIGHT; y++) {
for (int x = 0; x < WIDTH; x++) {
printf("%c", maze[y][x]);
}
printf("\n");
}
}
int main() {
int playerX = 1, playerY = 1;
char userInput = '\0';
drawMaze(playerX, playerY);
while (userInput != 'q') {
userInput = getch();
int newPlayerX = playerX;
int newPlayerY = playerY;
switch (userInput) {
case 'w':
newPlayerY = playerY - 1;
break;
case 'a':
newPlayerX = playerX - 1;
break;
case 's':
newPlayerY = playerY + 1;
break;
case 'd':
newPlayerX = playerX + 1;
break;
}
if (maze[newPlayerY][newPlayerX] == ' ') {
playerX = newPlayerX;
playerY = newPlayerY;
}
drawMaze(playerX, playerY);
}
return 0;
}
```
这个代码示例创建了一个迷宫的字符数组,并使用`drawMaze`函数在控制台上打印迷宫。`playerX`和`playerY`变量表示玩家在迷宫中的位置。通过接收用户输入的字符来移动玩家,按下'w'向上移动,按下'a'向左移动,按下's'向下移动,按下'd'向右移动。当按下'q'时,游戏退出。
代码中使用了`getch`函数来接收并获取用户的按键输入,`system("cls")`函数来清空屏幕并显示新的迷宫状态。
请注意,此代码只是一个简单的迷宫游戏示例,可能没有过多的功能和复杂性。您可以根据自己的需求来扩展和完善它。
阅读全文
相关推荐
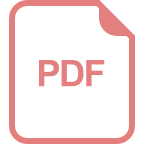
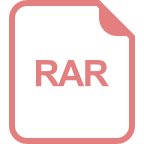
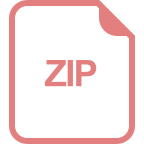
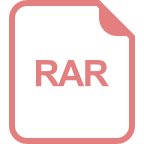
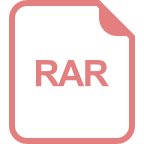
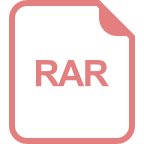
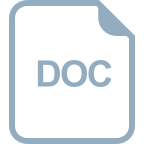

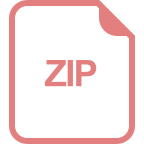
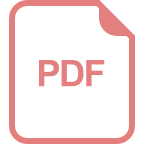
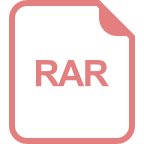
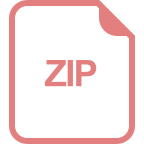
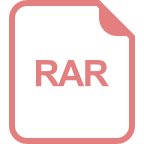
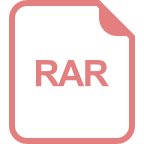
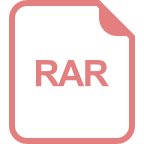
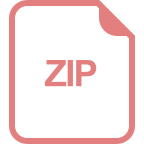
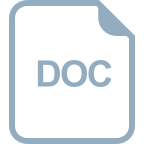
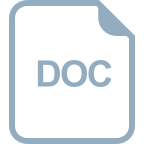