用C++写一个带有存取款,计算利息的银行管理系统
时间: 2024-04-23 18:24:24 浏览: 73
好的,这里是一个带有存取款、计算利息和查询余额的银行管理系统的C++代码示例,仅供参考:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Account {
private:
string name;
string accountNumber;
double balance;
double interestRate;
public:
// 构造函数
Account(string name, string accountNumber, double balance, double interestRate) {
this->name = name;
this->accountNumber = accountNumber;
this->balance = balance;
this->interestRate = interestRate;
}
// 存款
void deposit(double amount) {
balance += amount;
}
// 取款
bool withdraw(double amount) {
if (balance < amount) {
return false;
} else {
balance -= amount;
return true;
}
}
// 计算利息
double calculateInterest() {
return balance * interestRate;
}
// 查询余额
double getBalance() {
return balance;
}
// 获取账户信息
void getAccountInfo() {
cout << "账户名:" << name << endl;
cout << "账号:" << accountNumber << endl;
cout << "余额:" << balance << endl;
cout << "利率:" << interestRate << endl;
}
};
int main() {
// 创建一个账户
Account account("张三", "10001", 1000.0, 0.01);
// 存款
account.deposit(1000.0);
cout << "存款成功!" << endl;
// 取款
bool withdrawResult = account.withdraw(500.0);
if (withdrawResult) {
cout << "取款成功!" << endl;
} else {
cout << "余额不足,取款失败。" << endl;
}
// 计算利息
double interest = account.calculateInterest();
cout << "本月利息为:" << interest << endl;
// 查询余额
cout << "当前余额为:" << account.getBalance() << endl;
return 0;
}
```
在这段代码中,我们新增了一个计算利息的功能,并且将 accountNumber 类型改为了字符串类型,以支持更多的账号格式。在 main 函数中,我们创建了一个账户,进行了存款、取款、计算利息和查询余额等操作,并输出了相应的结果。需要注意的是,这个代码仅是一个简单的示例,实际开发中需要考虑更多的业务逻辑和安全性问题。
阅读全文
相关推荐

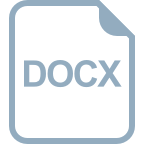
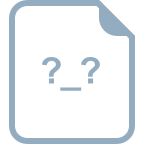
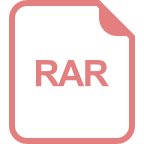
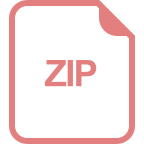
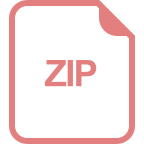
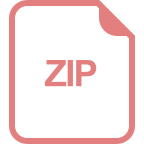
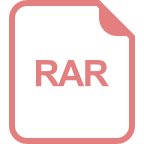
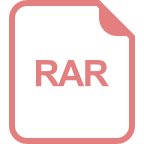
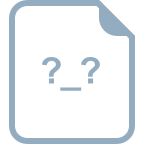
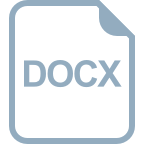