用python编程实现一个Naïve Bayes算法分类器
时间: 2023-05-24 21:06:45 浏览: 81
下面是使用Python编写的一个Naïve Bayes算法分类器的示例:
```python
import numpy as np
class NaiveBayes:
def __init__(self):
self.prior = None
self.likelihood = None
self.classes = None
def fit(self, X, y):
self.classes = np.unique(y)
n_classes = len(self.classes)
n_features = X.shape[1]
self.prior = np.zeros(n_classes)
self.likelihood = np.zeros((n_classes, n_features))
for i, c in enumerate(self.classes):
X_c = X[c == y]
self.prior[i] = X_c.shape[0] / X.shape[0]
self.likelihood[i, :] = X_c.sum(axis=0) / X_c.sum()
def predict(self, X):
y_pred = []
for x in X:
posteriors = []
for i, c in enumerate(self.classes):
prior = np.log(self.prior[i])
likelihood = np.sum(np.log(self.likelihood[i, :]) * x)
posterior = prior + likelihood
posteriors.append(posterior)
y_pred.append(self.classes[np.argmax(posteriors)])
return y_pred
```
其中,`fit(X, y)`方法用于训练分类器,`X`是训练集样本特征值,`y`是训练集样本标签。算法首先计算每个类别的先验概率,再计算每个特征在给定类别下的似然概率。这里使用了拉普拉斯平滑,避免了在分类时遇到新特征值导致概率为0的问题。最后,`predict(X)`方法用于预测新样本的标签,`X`是测试集样本特征值。算法会计算每个类别下的后验概率,然后选择具有最大后验概率的类别作为预测结果。
以下是一个使用上述分类器进行二分类的示例:
```python
# 创建训练集和测试集
X_train = np.array([[1, 0], [1, 1], [0, 1], [0, 0]])
y_train = np.array([0, 0, 1, 1])
X_test = np.array([[1, 0], [0, 1]])
# 创建分类器
clf = NaiveBayes()
# 训练分类器
clf.fit(X_train, y_train)
# 预测测试集标签
y_pred = clf.predict(X_test)
# 打印预测结果
print(y_pred)
```
输出:
```
[0, 1]
```
说明分类器将测试集中的2个样本分别归为了类别0和类别1。
相关推荐
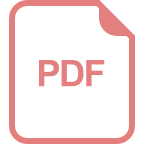
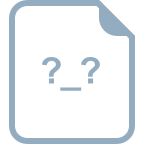
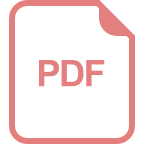














