数据结构C语言版线性结构代码
时间: 2024-10-11 17:06:13 浏览: 24
在C语言中,线性数据结构主要用于存储和组织元素,通常按照一种顺序的方式排列。以下是几个常见的C语言线性数据结构及其代码示例:
1. **数组**:
```c
typedef struct {
int size;
int* data; // 存储元素的指针
} Array;
// 初始化数组
Array* createArray(int capacity) {
Array* arr = (Array*)malloc(sizeof(Array));
arr->size = capacity;
arr->data = (int*)malloc(capacity * sizeof(int));
return arr;
}
// 添加元素
void insertArray(Array* arr, int value, int index) {
if (index < arr->size) {
arr->data[index] = value;
} else {
printf("Index out of range.\n");
}
}
// 其他操作如删除、遍历等...
```
2. **链表**:
```c
typedef struct Node {
int value;
struct Node* next;
} Node;
// 链表头结点
struct List {
Node* head;
};
// 创建链表
List* createList() {
List* list = (List*)malloc(sizeof(List));
list->head = NULL;
return list;
}
// 插入节点
void insertNode(List* list, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->value = value;
newNode->next = list->head;
list->head = newNode;
}
```
阅读全文
相关推荐
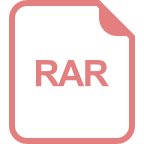
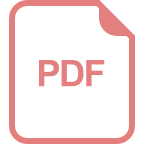
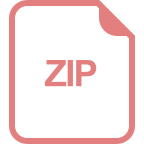
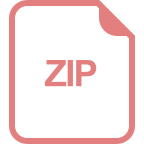
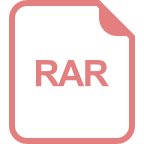
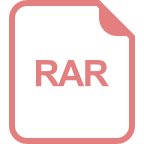
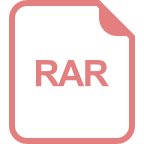
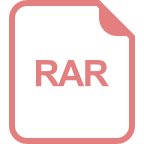
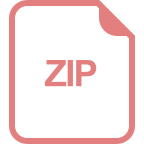
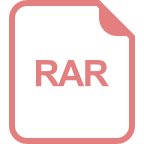
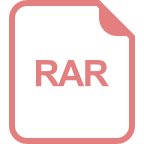
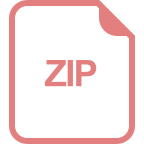
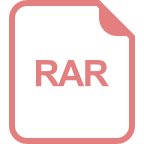
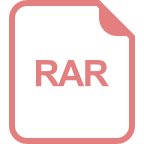
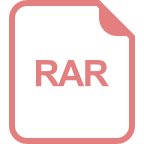
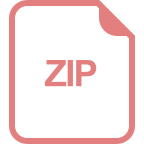
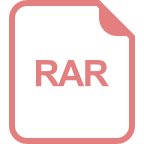
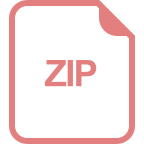