Qt中实现队列元素在label中依次出现并停留在最后一个元素
时间: 2024-10-15 13:24:41 浏览: 37
在Qt中,要实现队列元素逐个显示并在Label(通常指QLabel)上停留,你可以使用`QTimer`配合`QQueue`来创建一个动画效果。这里是一个基本步骤:
1. 创建一个`QQueue<QString>`用于存放队列元素。
2. 使用`QTimer`设置定时器,每隔一段时间从队列中取出一个元素,并更新Label的内容。
3. 当队列为空时,停止定时器。
下面是一个简单的示例代码,假设我们有一个队列`queue`,一个` QLabel *label` 和一个定时器`timer`:
```cpp
#include <QObject>
#include <QQueue>
#include <QTimer>
#include <QLabel>
class QLabelWithQueue : public QObject {
public:
QLabelWithQueue(QQueue<QString> queue, QLabel *label)
: queue_(queue), label_(label) {
// 初始化定时器
timer_ = new QTimer(this);
connect(timer_, &QTimer::timeout, this, [this]() {
if (!queue_.isEmpty()) {
QString element = queue_.front();
label_->setText(element); // 更新Label内容
queue_.pop(); // 移除第一个元素
// 如果队列还剩下元素,设置下一个计时时间
if (!queue_.isEmpty())
timer_->start(interval_);
} else { // 队列空了,关闭定时器
timer_->stop();
}
});
// 设置初始间隔时间(例如1秒)
interval_ = 1000;
startTimer(); // 开始定时器
}
private:
QQueue<QString> queue_;
QLabel *label_;
QTimer *timer_;
int interval_; // 定时器间隔
void startTimer() {
if (!queue_.isEmpty())
timer_->start(interval_);
}
};
```
在这个例子中,你需要创建一个`QLabelWithQueue`实例,并传入队列和Label的引用。然后,队列中的每个元素都会按照设定的时间间隔轮流显示在Label上,直到队列为空。
阅读全文
相关推荐
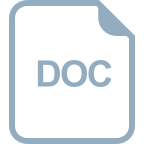
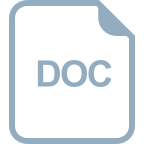
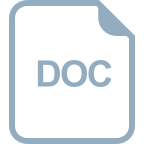
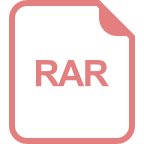
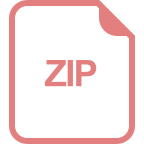
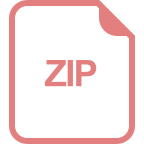
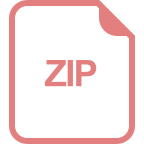
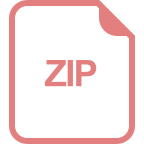
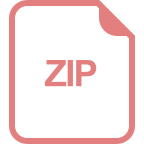
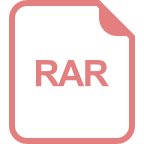
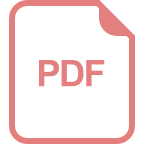
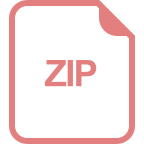
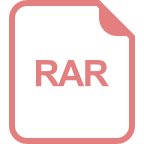
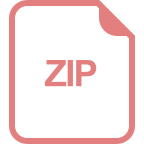
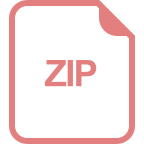
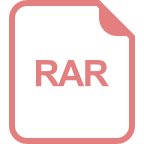
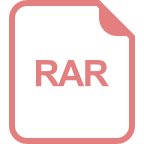
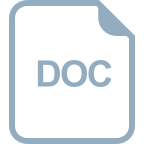