帮我写一个java获取word、ppt、pdf、excel等文档页数的工具类
时间: 2023-07-10 11:26:22 浏览: 210
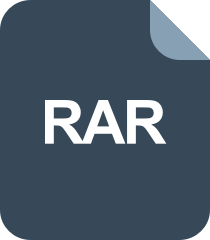
Java读取Word文档页数
好的,以下是一个Java获取各种文档页数的工具类,使用了Apache POI和Apache PDFBox库:
```
import java.io.File;
import java.io.FileInputStream;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.apache.poi.hslf.usermodel.HSLFSlideShow;
import org.apache.poi.sl.usermodel.SlideShow;
import org.apache.poi.hwpf.HWPFDocument;
import org.apache.poi.hwpf.usermodel.Range;
import org.apache.poi.xslf.usermodel.XMLSlideShow;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.pdfbox.pdmodel.PDDocument;
public class DocumentPageCount {
public static int getExcelPageCount(String filePath) {
try {
File file = new File(filePath);
FileInputStream fis = new FileInputStream(file);
Workbook workbook = null;
if (filePath.endsWith(".xls")) {
workbook = new HSSFWorkbook(fis);
} else if (filePath.endsWith(".xlsx")) {
workbook = new XSSFWorkbook(fis);
}
fis.close();
return workbook.getNumberOfSheets();
} catch (Exception e) {
e.printStackTrace();
return 0;
}
}
public static int getPptPageCount(String filePath) {
try {
File file = new File(filePath);
FileInputStream fis = new FileInputStream(file);
SlideShow slideshow = null;
if (filePath.endsWith(".ppt")) {
slideshow = new HSLFSlideShow(fis);
} else if (filePath.endsWith(".pptx")) {
slideshow = new XMLSlideShow(fis);
}
fis.close();
return slideshow.getSlides().size();
} catch (Exception e) {
e.printStackTrace();
return 0;
}
}
public static int getWordPageCount(String filePath) {
try {
File file = new File(filePath);
FileInputStream fis = new FileInputStream(file);
HWPFDocument doc = null;
Range range = null;
XWPFDocument docx = null;
if (filePath.endsWith(".doc")) {
doc = new HWPFDocument(fis);
range = doc.getRange();
} else if (filePath.endsWith(".docx")) {
docx = new XWPFDocument(fis);
range = docx.getDocument().getBody().getDocumentContent().getEndOfContent().getRange();
}
fis.close();
return range.numParagraphs();
} catch (Exception e) {
e.printStackTrace();
return 0;
}
}
public static int getPdfPageCount(String filePath) {
try {
File file = new File(filePath);
PDDocument document = PDDocument.load(file);
int pageCount = document.getNumberOfPages();
document.close();
return pageCount;
} catch (Exception e) {
e.printStackTrace();
return 0;
}
}
}
```
这个工具类包含了四个方法,分别用于获取Excel、PPT、Word和PDF文档的页数。您可以在自己的项目中使用这些方法来获取文档的页数。例如,要获取一个Excel文件的页数,可以调用`DocumentPageCount.getExcelPageCount("path/to/excel/file.xls")`。
阅读全文
相关推荐
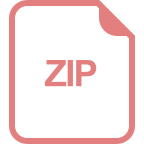
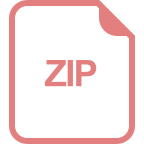
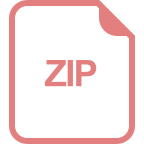
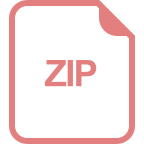
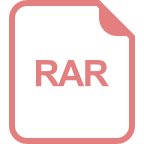
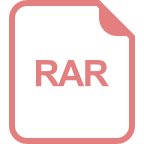
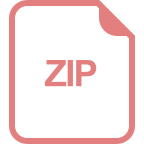
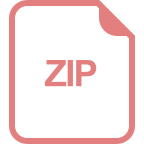
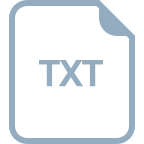
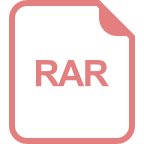
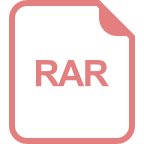
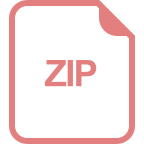
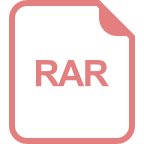
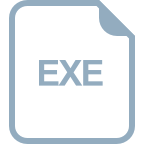
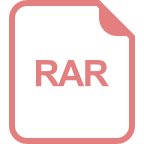