Linux之线程同步二第三关项目实战代码
时间: 2024-01-16 13:03:18 浏览: 29
以下是一个简单的线程同步示例代码,使用了互斥锁和条件变量来实现线程的同步。
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define BUFFER_SIZE 10
int buffer[BUFFER_SIZE];
int count = 0;
pthread_mutex_t mutex;
pthread_cond_t full, empty;
void *producer(void *arg) {
int item;
while (1) {
item = rand() % 100; // 随机生成一个数字作为生产的物品
pthread_mutex_lock(&mutex);
while (count == BUFFER_SIZE) { // 缓冲区满了,等待消费者消费
pthread_cond_wait(&empty, &mutex);
}
buffer[count++] = item; // 放入缓冲区
printf("Produced item %d\n", item);
pthread_mutex_unlock(&mutex);
pthread_cond_signal(&full); // 唤醒消费者
sleep(rand() % 3); // 生产完等待一段时间再生产
}
}
void *consumer(void *arg) {
int item;
while (1) {
pthread_mutex_lock(&mutex);
while (count == 0) { // 缓冲区空了,等待生产者生产
pthread_cond_wait(&full, &mutex);
}
item = buffer[--count]; // 从缓冲区取出物品
printf("Consumed item %d\n", item);
pthread_mutex_unlock(&mutex);
pthread_cond_signal(&empty); // 唤醒生产者
sleep(rand() % 3); // 消费完等待一段时间再消费
}
}
int main() {
pthread_t tid1, tid2;
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&full, NULL);
pthread_cond_init(&empty, NULL);
pthread_create(&tid1, NULL, producer, NULL);
pthread_create(&tid2, NULL, consumer, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&full);
pthread_cond_destroy(&empty);
return 0;
}
```
在这个示例中,有一个线程作为生产者,随机生成数字作为物品,并将物品放入缓冲区中;另一个线程作为消费者,从缓冲区中取出物品。生产者和消费者之间通过互斥锁和条件变量来进行同步,保证线程的安全性和正确性。
相关推荐
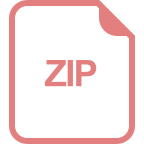
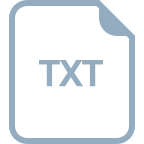
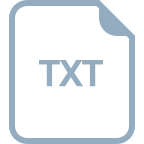














