写一段java实现的邮件收发系统
时间: 2023-11-23 07:06:59 浏览: 117
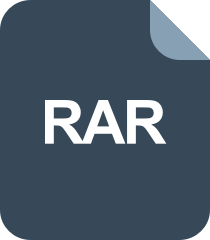
java写的邮件收发系统

好的,以下是一段简单的 Java 代码实现邮件收发系统:
```
import java.util.Properties;
import javax.mail.*;
import javax.mail.internet.*;
public class EmailSystem {
public static void main(String[] args) {
final String username = "your-email-address@gmail.com";
final String password = "your-email-password";
Properties props = new Properties();
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.host", "smtp.gmail.com");
props.put("mail.smtp.port", "587");
Session session = Session.getInstance(props, new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
});
try {
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress("your-email-address@gmail.com"));
message.setRecipients(
Message.RecipientType.TO,
InternetAddress.parse("recipient-email-address@other.com")
);
message.setSubject("Testing Subject");
message.setText("Dear Recipient,\n\nThis is a test email. Cheers!");
Transport.send(message);
System.out.println("Email sent!");
} catch (MessagingException e) {
throw new RuntimeException(e);
}
}
}
```
这些代码让你使用 Gmail 的 SMTP 服务器发送邮件。你可以把 `your-email-address` 和 `your-email-password` 替换为你自己的 Gmail 账户的地址和密码,然后在 `InternetAddress.parse` 中指定收件人地址。这段代码只是一个示例,你可以根据自己的需求进行修改。
阅读全文
相关推荐
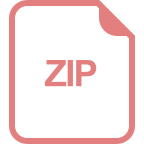
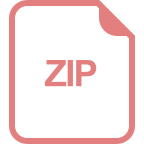
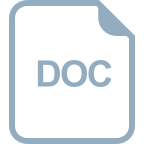
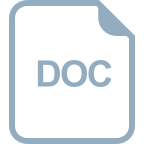
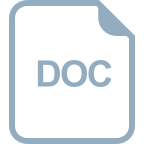
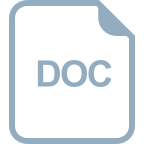
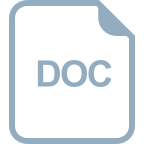
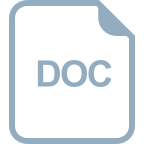
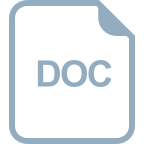
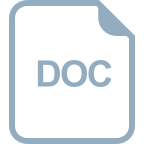
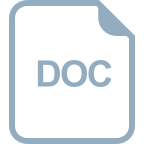
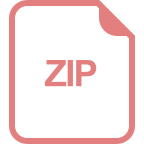
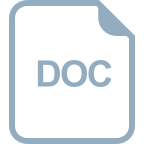
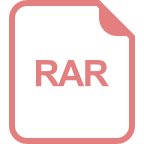
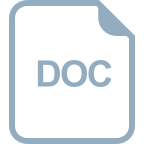
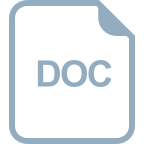
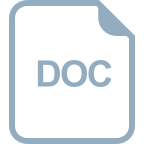