Unity获取系统详细信息
时间: 2024-05-09 22:16:02 浏览: 7
Unity可以使用SystemInfo类来获取系统的详细信息,包括操作系统版本、CPU型号、GPU型号、内存大小等。以下是一些示例代码:
```csharp
using UnityEngine;
public class SystemInfoExample : MonoBehaviour
{
void Start()
{
Debug.Log("Operating System: " + SystemInfo.operatingSystem);
Debug.Log("CPU: " + SystemInfo.processorType);
Debug.Log("GPU: " + SystemInfo.graphicsDeviceName);
Debug.Log("System Memory Size: " + SystemInfo.systemMemorySize + "MB");
}
}
```
在Unity的编辑器中运行此代码将在控制台中输出系统信息。注意,某些信息可能无法在所有平台上获得。例如,某些移动设备可能没有详细的CPU或GPU信息。
相关问题
Unity3d获取windows系统的原始输入数据
在Unity3D中获取Windows系统的原始输入数据,可以使用`RawInput.NET`插件。以下是使用该插件的示例代码:
1. 下载并导入`RawInput.NET`插件到Unity项目中。
2. 创建一个C#脚本,比如命名为`RawInputExample.cs`,并将以下代码添加到脚本中:
```csharp
using UnityEngine;
using RawInput;
public class RawInputExample : MonoBehaviour
{
private void Start()
{
RawKeyInput.Start();
RawMouseInput.Start();
}
private void Update()
{
while (RawKeyInput.HasMessages())
{
RawKeyInputMessage message = RawKeyInput.GetMessage();
Debug.Log("Key: " + message.VKey);
Debug.Log("Flags: " + message.Flags);
Debug.Log("MakeCode: " + message.MakeCode);
}
while (RawMouseInput.HasMessages())
{
RawMouseInputMessage message = RawMouseInput.GetMessage();
Debug.Log("X: " + message.X);
Debug.Log("Y: " + message.Y);
Debug.Log("WheelDelta: " + message.WheelDelta);
}
}
private void OnApplicationQuit()
{
RawKeyInput.Stop();
RawMouseInput.Stop();
}
}
```
3. 在Unity编辑器中,将该脚本附加到一个物体上(比如一个空物体)。
4. 运行Unity项目,并在控制台中查看原始输入数据。
这样,你就可以通过使用`RawInput.NET`插件在Unity3D中获取Windows系统的原始输入数据了。注意,该插件只能在Windows平台上使用。
unity获取系统时间
在Unity中,可以使用C#中的`DateTime.Now`来获取当前系统时间。具体实现方法如下:
```C#
using UnityEngine;
using System;
public class GetSystemTime : MonoBehaviour
{
void Start()
{
DateTime now = DateTime.Now;
Debug.Log("当前系统时间:" + now.ToString());
}
}
```
这样就可以获取当前系统时间并在控制台中输出了。需要注意的是,`DateTime.Now`返回的是本地时间,如果需要获取标准时间,可以使用`DateTime.UtcNow`。
相关推荐
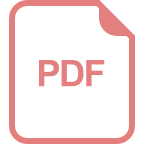












