springboot 获取Session Storage的key
时间: 2024-02-17 16:01:31 浏览: 140
在Spring Boot中,可以通过以下方式获取Session Storage的key:
```java
@GetMapping("/get-session-key")
public String getSessionKey(HttpServletRequest request) {
HttpSession session = request.getSession();
String sessionKey = session.getId(); // 获取Session ID,也就是存储在Session Storage中的key
return sessionKey;
}
```
在上面的代码中,我们首先获取HttpServletRequest对象,然后从中获取HttpSession对象。HttpSession对象包含了Session Storage中存储的所有数据,而Session ID就是用来唯一标识这个Session的key。因此,我们只需要调用`session.getId()`方法就可以获取Session ID了。
相关问题
springboot 获取页面 Session Storage 中的key
在Spring Boot中,我们可以通过前端代码获取Session Storage中的key,然后将其发送到后端进行处理。以下是一个示例:
```javascript
// 获取Session Storage中的key
var sessionKey = window.sessionStorage.key(index);
// 发送请求到后端
$.ajax({
url: '/get-session-value',
type: 'GET',
data: {
sessionKey: sessionKey
},
success: function(response) {
// 处理响应数据
console.log(response);
}
});
```
在上面的代码中,我们首先通过`window.sessionStorage.key(index)`方法获取Session Storage中指定位置的key。然后,我们可以通过AJAX发送一个GET请求到后端,将获取到的key作为参数传递过去。在后端,我们可以通过以下方式获取对应key的值:
```java
@GetMapping("/get-session-value")
public String getSessionValue(HttpServletRequest request, @RequestParam("sessionKey") String sessionKey) {
HttpSession session = request.getSession();
String sessionValue = (String) session.getAttribute(sessionKey); // 获取Session Storage中指定key的值
return sessionValue;
}
```
在上面的代码中,我们首先获取HttpServletRequest对象和Session对象。然后,我们可以通过`session.getAttribute(sessionKey)`方法获取Session Storage中指定key的值。最后,我们将获取到的值返回给前端。
springboot获取session
在 Spring Boot 中获取 HttpSession 对象可以通过 HttpServletRequest 的 getSession() 方法来实现。
例如:
```java
@Controller
public class MyController {
@RequestMapping("/test")
public String test(HttpServletRequest request) {
HttpSession session = request.getSession();
// 使用 session
return "success";
}
}
```
也可以使用 @Autowired 注入 HttpSession,例如:
```java
@Controller
public class MyController {
@Autowired
private HttpSession session;
@RequestMapping("/test")
public String test() {
// 使用 session
return "success";
}
}
```
当然还有另外一种方式,使用Spring MVC提供的 @SessionAttributes 注解来获取session
```
@Controller
@SessionAttributes("user")
public class MyController {
@RequestMapping("/test")
public String test(@ModelAttribute("user") User user) {
// 使用 user
return "success";
}
}
```
注意:使用 @SessionAttributes 注解时需要在对应的配置类中开启注解支持。
```
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Override
public void addArgumentResolvers(List<HandlerMethodArgumentResolver> resolvers) {
resolvers.add(new SessionAttributeMethodArgumentResolver());
}
}
```
阅读全文
相关推荐
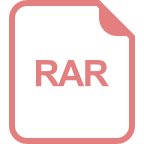
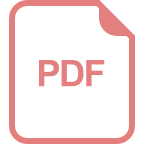

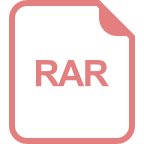











