写一函数,实现2个字符串的连接,函数形参用指针变量。例如"abcd"和"e12345",2个字符串,字符串的连接的结果为:"abcde12345"。
时间: 2023-06-09 19:05:14 浏览: 65
好的,您可以尝试使用以下的C语言函数:
```c
void stringConcat(char *str1, char *str2) {
while (*str1 != '\0') {
str1++;
}
while (*str2 != '\0') {
*str1 = *str2;
str1++;
str2++;
}
*str1 = '\0';
}
```
这个函数通过指针变量操作字符数组,将两个字符串连接在一起,最终结果存在第一个字符串数组中。您可以如下调用该函数:
```c
int main() {
char str1[100] = "abcd";
char str2[] = "e12345";
stringConcat(str1, str2);
printf("连接后的字符串为:%s\n", str1);
return 0;
}
```
输出结果为:
```
连接后的字符串为:abcde12345
```
相关问题
用c写一函数,实现2个字符串的连接,函数形参用指针变量。例如"abcd"和"e12345",2个字符串,字符串的连接的结果为:"abcde12345"。
好的,这是一个比较简单的问题,以下是用 C 语言编写的函数代码:
```c
#include <stdio.h>
#include <string.h>
void strAppend(char *s1, char *s2) {
strcat(s1, s2);
}
int main() {
char str1[100] = "abcd";
char str2[100] = "e12345";
strAppend(str1, str2);
printf("连接后的字符串为:%s\n", str1);
return 0;
}
```
函数 `strAppend` 接收两个字符串指针变量 `s1` 和 `s2`,使用 `strcat` 函数把两个字符串连接起来。在主函数中,我们定义两个字符串数组 `str1` 和 `str2`,并将字符串 "abcd" 和 "e12345" 存入。然后调用 `strAppend` 函数,把 `str2` 追加到 `str1` 末尾。最后输出 `str1` 即为连接后的字符串。
用c语言写一函数,实现2个字符串的连接,函数形参用指针变量。例如"abcd"和"e12345",2个字符串,字符串的连接的结果为:"abcde12345"。
好的,这是一个关于C语言函数的问题。下面是一个示例函数,可以实现两个字符串的连接:
```c
void string_concat(char *str1, char *str2) {
while (*str1 != '\0') { // 遍历第一个字符串
str1++;
}
while (*str2 != '\0') { // 将第二个字符串接到第一个字符串的末尾
*str1 = *str2;
str1++;
str2++;
}
*str1 = '\0'; // 最后需要添加字符串结束符
}
```
调用示例函数:
```c
char str1[] = "abcd";
char str2[] = "e12345";
string_concat(str1, str2);
printf("%s", str1);
```
输出结果为:
```
abcde12345
```
相关推荐
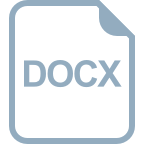
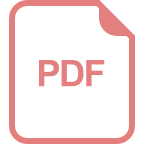
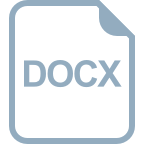












