List<Vector3> points赋值
时间: 2023-07-08 22:08:26 浏览: 58
要给List<Vector3>类型的变量points赋值,可以使用以下代码:
```csharp
List<Vector3> points = new List<Vector3>();
points.Add(new Vector3(0, 0, 0));
points.Add(new Vector3(1, 0, 0));
points.Add(new Vector3(0, 1, 0));
points.Add(new Vector3(0, 0, 1));
```
这段代码会创建一个名为points的List<Vector3>对象,并且给它添加了四个Vector3类型的元素,分别是(0, 0, 0)、(1, 0, 0)、(0, 1, 0)和(0, 0, 1)。你也可以使用其他方法向List<Vector3>对象添加元素,例如Insert()方法、AddRange()方法等。
相关问题
public List<Vector3> points;如何初始化
你可以通过以下方式之一来初始化 List<Vector3> 类型的变量 points:
1. 在声明变量时初始化:
```
List<Vector3> points = new List<Vector3> { new Vector3(0, 0, 0), new Vector3(1, 1, 1), new Vector3(2, 2, 2) };
```
2. 使用 Add 方法添加元素:
```
List<Vector3> points = new List<Vector3>();
points.Add(new Vector3(0, 0, 0));
points.Add(new Vector3(1, 1, 1));
points.Add(new Vector3(2, 2, 2));
```
3. 使用数组转换为 List:
```
Vector3[] pointArray = new Vector3[] { new Vector3(0, 0, 0), new Vector3(1, 1, 1), new Vector3(2, 2, 2) };
List<Vector3> points = new List<Vector3>(pointArray);
```
public List<Vector3> points;如何清除
可以使用 `points.Clear()` 方法来清空 List 中的元素,将 List 的长度设置为 0。例如:
```
points.Clear();
```
这将清空 `points` List 中的所有元素。如果需要重新添加元素,可以使用 `points.Add()` 方法。
相关推荐
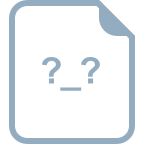
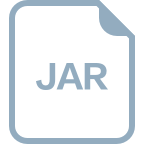












