基于vue2写一个画画的游戏代码
时间: 2024-05-10 09:17:08 浏览: 8
这里提供一个基于Vue2的简单画画游戏代码,实现了画笔颜色、粗细、橡皮擦、清空等功能。
```html
<template>
<div class="container">
<canvas ref="canvas" class="canvas"
@mousedown="startDrawing"
@mousemove="draw"
@mouseup="stopDrawing"
@mouseleave="stopDrawing"
></canvas>
<div class="tools">
<div class="colors">
<div class="color" v-for="(color, index) in colors" :key="index"
:style="{backgroundColor: color, border: (color === currentColor) ? '3px solid #000' : 'none'}"
@click="changeColor(color)"
></div>
</div>
<div class="sizes">
<div class="size" v-for="(size, index) in sizes" :key="index"
:style="{border: (size === currentSize) ? '3px solid #000' : 'none'}"
@click="changeSize(size)"
>{{ size }}</div>
</div>
<div class="actions">
<div class="erase" :class="{active: eraser}" @click="toggleEraser">橡皮擦</div>
<div class="clear" @click="clearCanvas">清空</div>
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
canvas: null, // 画布
ctx: null, // 画笔
isDrawing: false, // 是否正在画图
lastX: 0, // 上一个点的横坐标
lastY: 0, // 上一个点的纵坐标
currentColor: '#000', // 当前画笔颜色
colors: ['#000', '#f00', '#0f0', '#00f', '#ff0', '#f0f'], // 颜色列表
currentSize: '5', // 当前画笔粗细
sizes: ['1', '3', '5', '8', '10'], // 粗细列表
eraser: false, // 是否是橡皮擦
}
},
mounted() {
// 初始化画布
this.canvas = this.$refs.canvas
this.ctx = this.canvas.getContext('2d')
this.ctx.lineJoin = 'round'
this.ctx.lineCap = 'round'
},
methods: {
// 开始画图
startDrawing(e) {
this.isDrawing = true
this.lastX = e.offsetX
this.lastY = e.offsetY
},
// 继续画图
draw(e) {
if (!this.isDrawing) return
this.ctx.strokeStyle = this.eraser ? '#fff' : this.currentColor
this.ctx.lineWidth = this.currentSize
this.ctx.beginPath()
this.ctx.moveTo(this.lastX, this.lastY)
this.ctx.lineTo(e.offsetX, e.offsetY)
this.ctx.stroke()
this.lastX = e.offsetX
this.lastY = e.offsetY
},
// 停止画图
stopDrawing() {
this.isDrawing = false
},
// 改变画笔颜色
changeColor(color) {
this.currentColor = color
this.eraser = false
},
// 改变画笔粗细
changeSize(size) {
this.currentSize = size
},
// 切换为橡皮擦
toggleEraser() {
this.eraser = !this.eraser
},
// 清空画布
clearCanvas() {
this.ctx.clearRect(0, 0, this.canvas.width, this.canvas.height)
},
},
}
</script>
<style>
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.canvas {
border: 1px solid #000;
cursor: crosshair;
}
.tools {
display: flex;
flex-direction: column;
margin-left: 20px;
}
.colors {
display: flex;
margin-bottom: 20px;
}
.color {
width: 30px;
height: 30px;
margin-right: 10px;
cursor: pointer;
}
.sizes {
display: flex;
margin-bottom: 20px;
}
.size {
width: 30px;
height: 30px;
border-radius: 50%;
margin-right: 10px;
font-size: 14px;
display: flex;
justify-content: center;
align-items: center;
cursor: pointer;
}
.actions {
display: flex;
}
.erase, .clear {
border: 1px solid #000;
padding: 5px 10px;
margin-right: 10px;
cursor: pointer;
}
.erase.active {
background-color: #ccc;
}
</style>
```
在上面的代码中,我们使用了`canvas`元素来绘制画布,通过监听鼠标事件来实现画图功能,同时提供了改变画笔颜色、粗细、橡皮擦、清空等功能。注意在`mounted`生命周期函数中初始化画布和画笔,同时设置了`lineJoin`和`lineCap`属性来让线条更加平滑。
可以将以上代码保存为一个.vue文件,然后在Vue项目中引入即可。
相关推荐
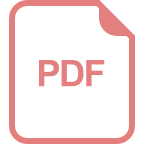
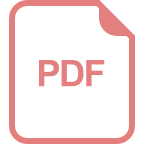















