c++中如何使用优先队列
时间: 2024-06-09 14:06:43 浏览: 160
在 C 语言中,可以使用优先队列来实现基于优先级的元素插入和删除操作。C 语言并没有内置的优先队列数据结构,但我们可以使用堆来实现优先队列。
首先,需要定义一个结构体来表示队列中的元素,该结构体包含优先级和数据两个成员变量。例如:
```c
typedef struct {
int priority; // 优先级
int data; // 数据
} Element;
```
然后,创建一个数组来保存队列中的元素。在插入和删除操作时,我们需要保持队列中元素的有序性,通常使用最小堆或最大堆来实现。在 C 语言中,可以使用数组来表示堆,并实现一些操作来维护堆的性质。
下面是一个简单的优先队列的实现示例:
```c
#include <stdio.h>
#define MAX_SIZE 100
typedef struct {
int priority;
int data;
} Element;
typedef struct {
Element heap[MAX_SIZE];
int size;
} PriorityQueue;
void enqueue(PriorityQueue* queue, Element element) {
if (queue->size >= MAX_SIZE) {
printf("Queue is full!\n");
return;
}
int index = queue->size;
while (index > 0 && element.priority < queue->heap[(index - 1) / 2].priority) {
queue->heap[index] = queue->heap[(index - 1) / 2];
index = (index - 1) / 2;
}
queue->heap[index] = element;
queue->size++;
}
Element dequeue(PriorityQueue* queue) {
if (queue->size <= 0) {
printf("Queue is empty!\n");
return (Element) {0, 0};
}
Element result = queue->heap[0];
Element lastElement = queue->heap[queue->size - 1];
int index = 0;
int childIndex = 1;
while (childIndex < queue->size) {
if (childIndex + 1 < queue->size && queue->heap[childIndex + 1].priority < queue->heap[childIndex].priority) {
childIndex++;
}
if (lastElement.priority < queue->heap[childIndex].priority) {
break;
}
queue->heap[index] = queue->heap[childIndex];
index = childIndex;
childIndex = childIndex * 2 + 1;
}
queue->heap[index] = lastElement;
queue->size--;
return result;
}
int main() {
PriorityQueue queue = {{0}, 0};
enqueue(&queue, (Element) {2, 20});
enqueue(&queue, (Element) {4, 40});
enqueue(&queue, (Element) {1, 10});
Element e1 = dequeue(&queue);
printf("Dequeued element: priority=%d data=%d\n", e1.priority, e1.data);
Element e2 = dequeue(&queue);
printf("Dequeued element: priority=%d data=%d\n", e2.priority, e2.data);
Element e3 = dequeue(&queue);
printf("Dequeued element: priority=%d data=%d\n", e3.priority, e3.data);
return 0;
}
```
在示例中,我们使用 `enqueue` 函数向优先队列中插入元素,使用 `dequeue` 函数从优先队列中删除并返回优先级最小的元素。在这个示例中,我们使用最小堆来实现优先队列。
阅读全文
相关推荐







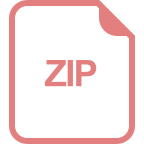

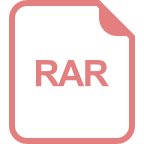






