C++使用protobuf流程
时间: 2023-09-04 08:15:03 浏览: 144
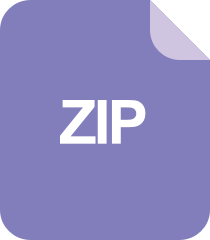
C++protobuf
使用protobuf的一般流程如下:
1. 定义消息格式:使用.proto文件定义消息格式,声明消息类型和字段。
2. 编写代码:使用protobuf提供的API,编写代码读写消息。
3. 编译.proto文件:使用protobuf的编译器protoc将.proto文件编译成C++代码。
4. 构建和运行程序:将生成的C++代码和编写的代码一起编译构建,并运行程序。
下面是一个简单的例子:
1. 定义消息格式,在example.proto文件中定义一个消息类型Person:
```
syntax = "proto3";
message Person {
int32 id = 1;
string name = 2;
repeated string email = 3;
}
```
2. 编写代码:
```
#include <iostream>
#include <fstream>
#include "example.pb.h"
using namespace std;
int main() {
// 创建一个Person对象
Person person;
person.set_id(123);
person.set_name("Alice");
person.add_email("alice@example.com");
person.add_email("alice@gmail.com");
// 将Person对象写入文件
fstream output("person.pb", ios::out | ios::binary);
if (!person.SerializeToOstream(&output)) {
cerr << "Failed to write person." << endl;
return -1;
}
// 从文件中读取Person对象
fstream input("person.pb", ios::in | ios::binary);
Person p;
if (!p.ParseFromIstream(&input)) {
cerr << "Failed to read person." << endl;
return -1;
}
// 打印Person对象的内容
cout << "id: " << p.id() << endl;
cout << "name: " << p.name() << endl;
for (int i = 0; i < p.email_size(); i++) {
cout << "email[" << i << "]: " << p.email(i) << endl;
}
return 0;
}
```
3. 编译.proto文件:
```
protoc --cpp_out=. example.proto
```
4. 构建和运行程序:
```
g++ -std=c++11 example.pb.cc main.cpp -lprotobuf -o main
./main
```
输出结果为:
```
id: 123
name: Alice
email[0]: alice@example.com
email[1]: alice@gmail.com
```
阅读全文
相关推荐

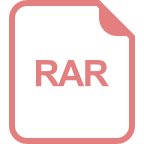
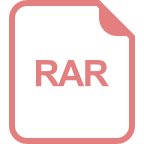
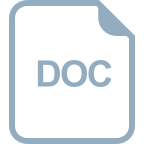
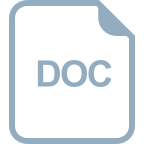
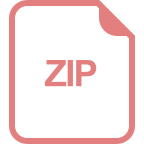
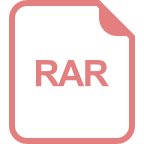
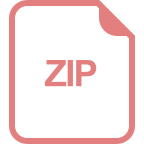
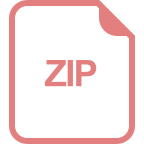
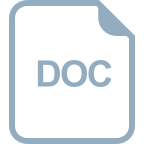
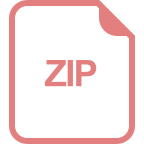
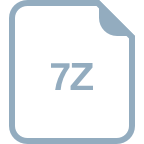
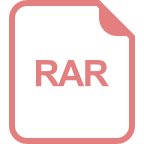
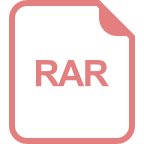
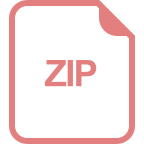
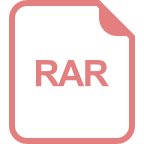