#define FUNC_EXIT_RC(x)
时间: 2024-06-23 19:01:31 浏览: 97
`#define FUNC_EXIT_RC(x)` 是一个预处理器宏定义(preprocessor macro),在C/C++编程中常用于简化错误处理或函数结束时的返回值管理。它的作用是在程序中定义一个简单的函数调用约定,其中 `x` 通常代表一个整数值,表示函数执行的结果或遇到的错误代码。
当在代码中使用这个宏时,它会被替换为一个等价于 `return x;` 的语句。这样做的好处是可以统一处理不同功能的函数在结束时返回相同类型的值,如错误码,而不需要每次都在函数体内部写 return 语句。例如:
```c
int main() {
int rc = FUNC_EXIT_RC(0); // 如果没有错误,rc就是0
if (rc != 0) {
// 处理错误
}
return rc;
}
// 使用宏的地方相当于:
int main() {
return 0; // 或者其他错误码
}
```
相关问题
#include <stdio.h> #include <stdlib.h> #include <pthread.h> #define NUM_THREADS 5 void *thread_func(void *thread_id) { long tid; tid = (long) thread_id; printf("Thread %ld is running\n", tid); // 创建一个新的线程 pthread_t sub_thread; int rc; rc = pthread_create(&sub_thread, NULL, thread_func, (void *) (tid + 1)); if (rc) { printf("Error: return code from pthread_create() is %d\n", rc); exit(-1); } pthread_exit(NULL); } int main(int argc, char *argv[]) { pthread_t threads[NUM_THREADS]; int rc; long t; for (t = 0; t < NUM_THREADS; t++) { printf("Creating thread %ld\n", t); rc = pthread_create(&threads[t], NULL, thread_func, (void *) t); if (rc) { printf("Error: return code from pthread_create() is %d\n", rc); exit(-1); } } // 等待所有线程结束 for (t = 0; t < NUM_THREADS; t++) { pthread_join(threads[t], NULL); } pthread_exit(NULL); }如何在第二个子线程里天机算法
您可以将您的算法代码放在`thread_func`函数中。在您创建新的子线程时,将`thread_func`作为参数传递给`pthread_create`函数,就可以在新的子线程中运行您的算法。以下是修改后的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 5
void *thread_func(void *thread_id) {
long tid;
tid = (long) thread_id;
printf("Thread %ld is running\n", tid);
// 在第二个子线程中添加算法
if (tid == 2) {
printf("Running algorithm in thread %ld\n", tid);
// 这里是您的算法代码
// ...
}
// 创建一个新的子线程
pthread_t sub_thread;
int rc;
rc = pthread_create(&sub_thread, NULL, thread_func, (void *) (tid + 1));
if (rc) {
printf("Error: return code from pthread_create() is %d\n", rc);
exit(-1);
}
pthread_exit(NULL);
}
int main(int argc, char *argv[]) {
pthread_t threads[NUM_THREADS];
int rc;
long t;
for (t = 0; t < NUM_THREADS; t++) {
printf("Creating thread %ld\n", t);
rc = pthread_create(&threads[t], NULL, thread_func, (void *) t);
if (rc) {
printf("Error: return code from pthread_create() is %d\n", rc);
exit(-1);
}
}
// 等待所有线程结束
for (t = 0; t < NUM_THREADS; t++) {
pthread_join(threads[t], NULL);
}
pthread_exit(NULL);
}
```
在函数`thread_func`中,当`tid`等于2时,您可以在该线程中运行您的算法。如果您需要访问共享资源,请考虑线程安全问题。
#include <stdio.h> #include <stdlib.h> #include <pthread.h> #include "jisuan.h" #include "shuru.h" #include "math.h" void *thread_func(void *thread_id); #define NUM_THREADS 2 int main(int argc, char *argv[]) { int rc; pthread_t threads[NUM_THREADS]; int ret; long t; shuru(); // for (t = 0; t < NUM_THREADS; t++) { printf("Creating thread %ld\n", t); rc = pthread_create(&threads[t], NULL, thread_func, (void *) t); if (rc) { printf("Error: return code from pthread_create() is %d\n", rc); exit(-1); } } for (t = 0; t < NUM_THREADS; t++) { pthread_join(threads[t], NULL); } pthread_exit(NULL); } void *thread_func(void *thread_id) { long tid; tid = (long) thread_id; printf("Thread %ld is running\n", tid); // if(tid == 0) { } if(tid == 1) { double h = 100.0; // 初始高度 double s = 0.0; // 总路程 // 计算落地路程 s = calculate_distance(h); // 输出结果 printf("第%d次落地时,球经过的总路程为:%.6f米\n", num, s); printf("第%d次反弹的高度为:%.6f米\n", num, h / pow(2, num)); } pthread_exit(NULL); }为什么不能正确传递参数num
在这段代码中,变量 num 没有被定义或初始化,所以在调用函数 calculate_distance() 和打印结果时会出现错误。要正确传递参数 num,需要在主函数中定义并初始化它,然后将它作为参数传递给 thread_func() 函数。例如:
```
int num = 10; // 初始化 num
pthread_create(&threads[t], NULL, thread_func, (void *) &num); // 将 num 的地址传递给线程函数
```
然后在 thread_func() 函数中需要使用指针来获取 num 的值,例如:
```
int *p_num = (int *) thread_id; // 将 thread_id 转换为 int 类型的指针
int num = *p_num; // 获取 num 的值
```
这样就可以在线程函数中正确使用 num 变量了。
阅读全文
相关推荐
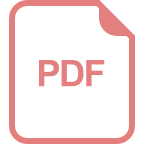
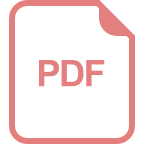
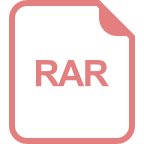

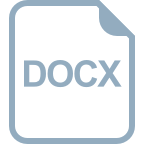
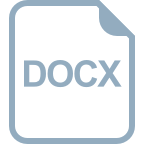
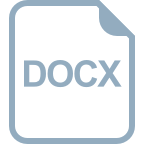
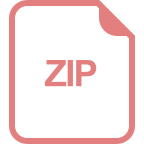