旅行商问题a星算法给出python代码实现
时间: 2024-12-18 15:13:56 浏览: 12
旅行商问题(Traveling Salesman Problem,TSP)是一个经典的组合优化问题,目的是寻找一条经过所有城市恰好一次并返回起点的最短路径。A*算法是一种启发式搜索算法,用于求解这类问题时通常可以找到相对接近最优解的解。
以下是Python中使用A*算法解决旅行商问题的一个简略示例:
```python
import heapq
# 定义节点类
class Node:
def __init__(self, city_id, cost_from_start, heuristic):
self.city_id = city_id
self.g_score = cost_from_start
self.h_score = heuristic(city_id)
self.f_score = self.g_score + self.h_score
self.previous = None
def __lt__(self, other):
return self.f_score < other.f_score
# A*算法核心函数
def a_star(tsp_cities, start_city):
open_list = [Node(start_city, 0, lambda x: tsp_cities.distances[start_city][x])] # 开放列表
closed_list = [] # 关闭列表
distances = {city: float('inf') for city in tsp_cities.cities} # 初始化距离字典
distances[start_city] = 0 # 设置起始点的距离为0
while open_list:
current_node = heapq.heappop(open_list) # 取f_score最小的城市
if current_node.city_id == tsp_cities.end_city: # 找到终点,返回路径
path = []
while current_node is not None:
path.append(current_node.city_id)
current_node = current_node.previous
path.append(start_city) # 添加起始点
path.reverse()
return path, current_node.g_score
closed_list.append(current_node)
for neighbor in tsp_cities.get_neighbors(current_node.city_id): # 遍历邻居
tentative_g_score = current_node.g_score + tsp_cities.distances[current_node.city_id][neighbor]
if tentative_g_score < distances[neighbor]:
distances[neighbor] = tentative_g_score
neighbor_node = Node(neighbor, tentative_g_score,
lambda x: tsp_cities.distances[neighbor][x])
neighbor_node.previous = current_node
if neighbor_node not in open_list:
heapq.heappush(open_list, neighbor_node)
# 假设你有一个TSPCity对象(包含城市列表、城市之间的距离等信息)
tsp_cities = ... # 实例化TSPCity
start_city = ... # 设定起始城市
solution_path, shortest_distance = a_star(tsp_cities, start_city)
print(f"最短路径: {solution_path}")
print(f"总距离: {shortest_distance}")
阅读全文
相关推荐
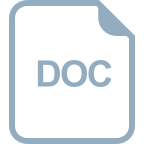
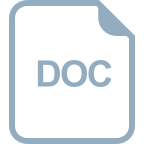
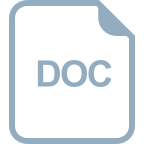
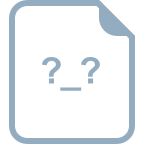
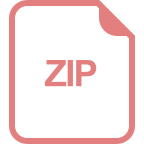
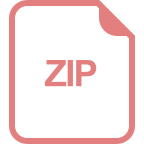
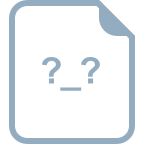
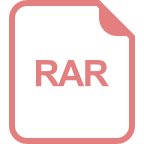
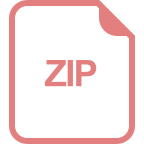
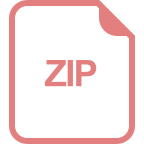
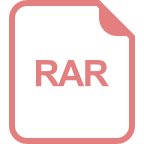
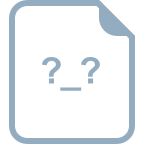
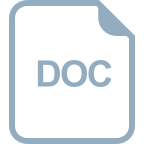
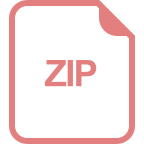
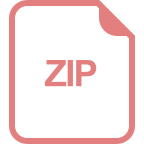