利用Python连接MySQL编写一个学生管理系统程序源代码
时间: 2024-02-27 09:55:54 浏览: 91
以下是一个简单的学生管理系统程序的源代码,利用Python连接MySQL数据库:
```python
import mysql.connector
# 连接MySQL数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="students"
)
# 创建游标对象
mycursor = mydb.cursor()
# 创建学生表
mycursor.execute("CREATE TABLE students (id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255), grade INT)")
# 添加学生记录
sql = "INSERT INTO students (name, grade) VALUES (%s, %s)"
val = ("John", 90)
mycursor.execute(sql, val)
# 添加多个学生记录
sql = "INSERT INTO students (name, grade) VALUES (%s, %s)"
val = [("Mike", 85), ("Sarah", 92), ("Tom", 88)]
mycursor.executemany(sql, val)
# 查询学生记录
mycursor.execute("SELECT * FROM students")
myresult = mycursor.fetchall()
for x in myresult:
print(x)
# 更新学生记录
sql = "UPDATE students SET grade = %s WHERE name = %s"
val = (95, "John")
mycursor.execute(sql, val)
# 删除学生记录
sql = "DELETE FROM students WHERE name = %s"
val = ("Tom",)
mycursor.execute(sql, val)
# 提交更改
mydb.commit()
# 关闭连接
mydb.close()
```
在这个学生管理系统程序中,我们使用Python的`mysql.connector`模块连接MySQL数据库,并进行了一些操作,如创建表、添加记录、查询记录、更新记录和删除记录。你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
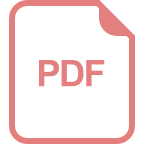
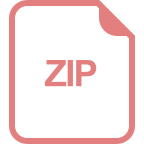
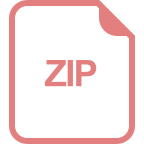
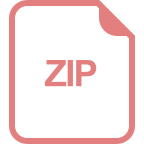
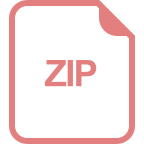
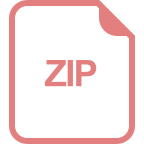
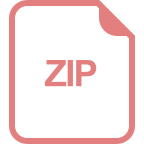
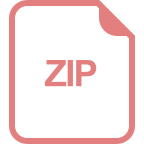
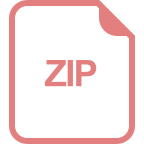
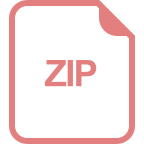
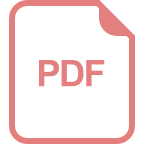
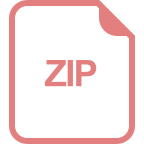
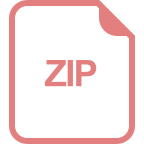
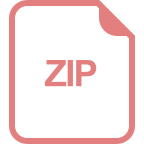
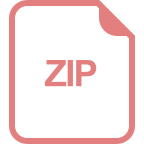
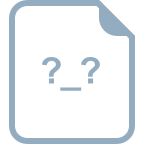
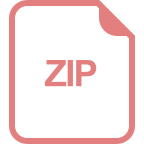
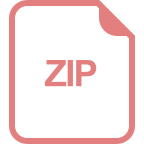