编写一个c语言多进程多线程的程序:要求创建4个子进程,每个子进程都分别创建两个线程,进程和线程的功能不作要求,可以只提供简单的打印语句。
时间: 2024-02-09 20:10:53 浏览: 83
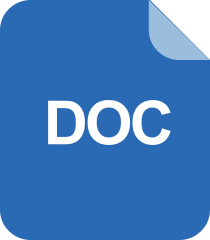
c语言多进程多线程编程
好的,下面是一个简单的多进程多线程程序的示例,满足你的要求:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <pthread.h>
#define NUM_PROCESSES 4
#define NUM_THREADS 2
void *thread_func(void *thread_id)
{
int tid = *((int*)thread_id);
printf("Thread %d is running in process %d\n", tid, getpid());
pthread_exit(NULL);
}
int main()
{
pid_t pid[NUM_PROCESSES];
pthread_t threads[NUM_PROCESSES][NUM_THREADS];
int process_ids[NUM_PROCESSES];
int thread_ids[NUM_THREADS];
int rc, t, p;
for (p = 0; p < NUM_PROCESSES; p++) {
printf("Creating process %d\n", p);
process_ids[p] = p;
pid[p] = fork();
if (pid[p] < 0) {
printf("ERROR: fork() failed\n");
exit(-1);
} else if (pid[p] == 0) {
// 子进程
printf("Child process %d is running\n", p);
for (t = 0; t < NUM_THREADS; t++) {
printf("Creating thread %d in process %d\n", t, getpid());
thread_ids[t] = t;
rc = pthread_create(&threads[p][t], NULL, thread_func, (void *)&thread_ids[t]);
if (rc){
printf("ERROR: return code from pthread_create() is %d\n", rc);
exit(-1);
}
}
// 等待所有线程结束
for (t = 0; t < NUM_THREADS; t++) {
pthread_join(threads[p][t], NULL);
}
printf("Child process %d ends\n", p);
exit(0);
}
}
// 等待所有子进程结束
for (p = 0; p < NUM_PROCESSES; p++) {
waitpid(pid[p], NULL, 0);
}
printf("All processes are done\n");
return 0;
}
```
这个程序中,主进程创建了 4 个子进程,并将它们的进程 ID 存储在数组 `pid` 中。每个子进程都会创建 2 个线程,其中每个线程执行 `thread_func` 函数,输出当前线程所在的进程 ID 和线程 ID。
程序最后等待所有子进程结束后,输出 "All processes are done"。
需要注意的是,多线程和多进程的编程模型和技术都比较复杂,在实际开发中需要仔细考虑各种可能的问题,比如进程间通信、同步和互斥、线程安全等等。因此,建议在需要使用多线程和多进程的场景下,认真学习相关的知识和技术,避免出现不必要的问题。
阅读全文
相关推荐
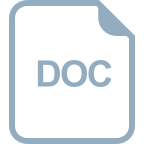
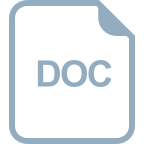
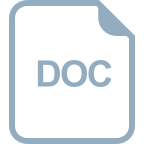
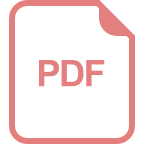
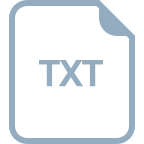
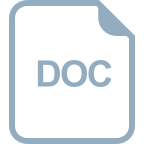
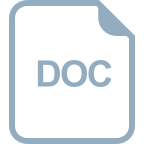
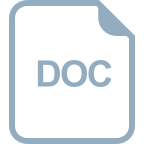
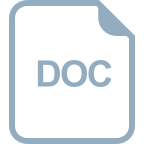
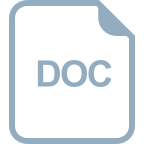
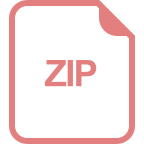
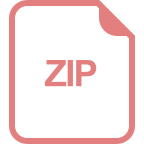
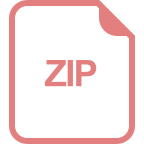
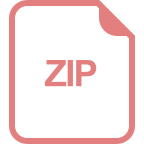
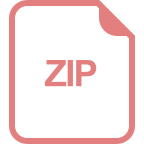
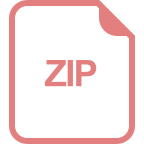