使用 CommonJS 的 require 语法来导入模块: 复制 const babylon = require('babylon');要下载module吗
时间: 2024-03-02 12:49:00 浏览: 150
是的,如果你想要使用 CommonJS 的 require 语法来导入模块,那么你需要先安装对应的模块,然后才能在代码中使用 require 导入该模块。
在你的示例代码中,使用了 require 导入了名为 babylon 的模块,因此你需要先使用 npm 下载 babylon 模块:
```
npm install babylon
```
然后在你的代码中使用 require 导入该模块:
```
const babylon = require('babylon');
```
这样就可以在你的代码中使用 babylon 模块了。请注意,使用 require 导入模块是 Node.js 中的一种常见方式,在浏览器环境中不一定适用。如果你需要在浏览器环境中使用模块化的方式导入模块,可以考虑使用 ES6 的 import 语法,并使用相关的构建工具进行转换和打包。
相关问题
CommonJS 导出使用:module.exports = {} CommonJS 导入使用:require(‘模块名或路径’) ECMAScript标准,命名导出为:export 修饰定义的语句 ECMAScript标准,命名导入为:import {同名变量} from ‘模块名或路径’
这些说法基本正确。
在 CommonJS 中,可以使用 `module.exports` 导出模块的内容,例如:
```javascript
// 定义模块
const value = 42;
const func = function() { /* ... */ }
module.exports = {
value,
func,
};
```
在导入时,可以使用 require 函数来导入其他模块的内容,例如:
```javascript
const otherModule = require('./otherModule.js');
console.log(otherModule.value);
otherModule.func();
```
在 ECMAScript 标准中,可以使用 `export` 修饰定义的语句来导出模块的内容,例如:
```javascript
// 定义模块
export const value = 42;
export function func() { /* ... */ }
```
在导入时,可以使用 `import` 命令来导入其他模块的内容,例如:
```javascript
import { value, func } from './otherModule.js';
console.log(value);
func();
```
需要注意的是,使用 `export` 导出的模块内容需要在导入时指定对应的名称,而不能像 CommonJS 那样直接访问整个模块对象。如果需要访问整个模块对象,可以使用 `import * as` 语法,例如:
```javascript
import * as otherModule from './otherModule.js';
console.log(otherModule.value);
otherModule.func();
```
我的代码:import { createRequire } from 'module'; const require = createRequire(import.meta.url); const os = require('os');,报错:Uncaught TypeError: Failed to resolve module specifier "module". Relative references must start with either "/", "./", or "../".
这个错误是因为您在使用 ES6 模块语法时,没有正确地导入模块。您需要在代码中使用 Node.js 的 CommonJS 模块语法。可以像这样修改您的代码:
```
const os = require('os');
```
这样您就可以正确地导入 `os` 模块并使用它了。
阅读全文
相关推荐
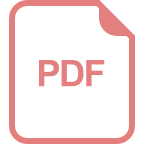
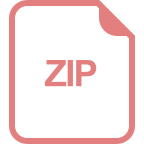
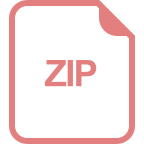
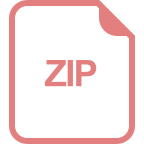
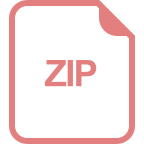
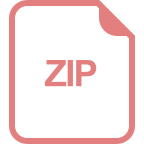
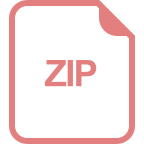
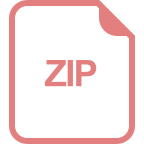
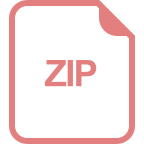
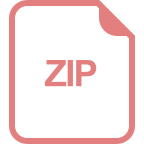
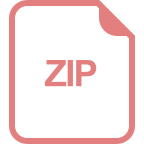
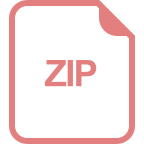
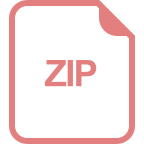
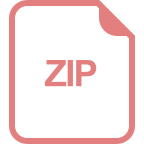
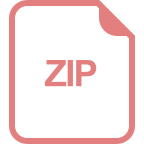
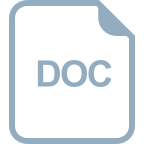
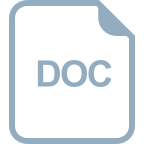