java调用graphql接口实现查询代码
时间: 2023-09-30 11:07:51 浏览: 51
假设我们有一个GraphQL接口,在其中定义了一个查询类型`query`,并且有一个名为`getUser`的查询字段,用于查询用户的详细信息。
下面是Java代码示例,用于调用该GraphQL接口并查询用户信息:
```java
import graphql.GraphQL;
import graphql.schema.DataFetchingEnvironment;
import graphql.schema.GraphQLObjectType;
import graphql.schema.GraphQLSchema;
import graphql.schema.StaticDataFetcher;
import graphql.schema.idl.RuntimeWiring;
import graphql.schema.idl.SchemaGenerator;
import graphql.schema.idl.SchemaParser;
import graphql.schema.idl.TypeDefinitionRegistry;
import java.io.File;
import java.io.IOException;
import java.util.Map;
import com.fasterxml.jackson.databind.ObjectMapper;
public class GraphQLClient {
public static void main(String[] args) throws IOException {
// 读取GraphQL schema定义
File schemaFile = new File("schema.graphqls");
String schemaString = FileUtils.readFileToString(schemaFile, "UTF-8");
// 创建GraphQL schema解析器
SchemaParser schemaParser = new SchemaParser();
TypeDefinitionRegistry typeDefinitionRegistry = schemaParser.parse(schemaString);
// 创建运行时绑定
RuntimeWiring runtimeWiring = RuntimeWiring.newRuntimeWiring()
.type("Query", builder -> builder
.dataFetcher("getUser", new StaticDataFetcher(Map.of(
"id", 1,
"name", "Alice",
"email", "alice@example.com"
)))
)
.build();
// 创建GraphQL schema生成器
SchemaGenerator schemaGenerator = new SchemaGenerator();
GraphQLSchema graphQLSchema = schemaGenerator.makeExecutableSchema(typeDefinitionRegistry, runtimeWiring);
// 创建GraphQL实例
GraphQL graphQL = GraphQL.newGraphQL(graphQLSchema).build();
// 定义查询语句
String query = "{ getUser { id name email } }";
// 执行查询
Map<String, Object> result = graphQL.execute(query).getData();
// 输出结果
ObjectMapper objectMapper = new ObjectMapper();
System.out.println(objectMapper.writeValueAsString(result));
}
}
```
在上面的代码中,我们首先读取GraphQL schema定义文件并解析它,然后创建运行时绑定以定义查询字段的数据抓取器。
接下来,我们使用GraphQL schema生成器创建实际的GraphQL schema,并使用它创建一个GraphQL实例来执行查询。
最后,我们定义查询语句,执行查询,并输出结果。在这个示例中,我们只查询了一个用户,但是你可以根据你的需要修改查询语句和数据抓取器来查询更多的信息。
相关推荐
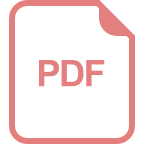














