写一份关于学生管理系统的python代码程序,运用到多线程
时间: 2023-04-07 22:00:43 浏览: 63
我可以回答这个问题。以下是一个简单的学生管理系统的Python代码程序,它使用了多线程来提高程序的效率:
```python
import threading
class Student:
def __init__(self, name, age, grade):
self.name = name
self.age = age
self.grade = grade
class StudentManager:
def __init__(self):
self.students = []
self.lock = threading.Lock()
def add_student(self, student):
with self.lock:
self.students.append(student)
def remove_student(self, student):
with self.lock:
self.students.remove(student)
def print_students(self):
with self.lock:
for student in self.students:
print(f"{student.name}, {student.age}, {student.grade}")
if __name__ == "__main__":
manager = StudentManager()
# Create some students
s1 = Student("Alice", 18, "A")
s2 = Student("Bob", 19, "B")
s3 = Student("Charlie", 20, "C")
# Add students to the manager
t1 = threading.Thread(target=manager.add_student, args=(s1,))
t2 = threading.Thread(target=manager.add_student, args=(s2,))
t3 = threading.Thread(target=manager.add_student, args=(s3,))
t1.start()
t2.start()
t3.start()
t1.join()
t2.join()
t3.join()
# Print the students
manager.print_students()
```
这个程序定义了一个`Student`类和一个`StudentManager`类。`Student`类表示一个学生,包含姓名、年龄和成绩三个属性。`StudentManager`类用于管理学生,包含添加、删除和打印学生信息等方法。为了保证线程安全,`StudentManager`类使用了一个锁来保护共享数据。
在`main`函数中,我们创建了三个学生对象,并使用多线程将它们添加到`StudentManager`中。最后,我们打印了所有学生的信息。
这个程序只是一个简单的示例,实际的学生管理系统可能需要更多的功能和更复杂的实现。但是,使用多线程可以提高程序的效率,让用户更快地完成操作。
相关推荐
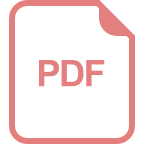
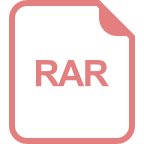
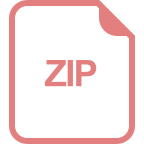














