基于STM32F103C8T6单片机写一段NEC解码程序,要求通过PB9引脚连接接收端,然后将解出的码通过PB8发送出去
时间: 2023-11-26 08:06:41 浏览: 145
以下是基于STM32F103C8T6单片机的NEC解码程序,需要通过PB9引脚连接接收端,然后将解出的码通过PB8发送出去:
```c
#include "stm32f10x.h"
void IR_Decode_Init(void);
void IR_Decode_GPIO_Config(void);
void IR_Decode_EXTI_Config(void);
void USART1_Config(void);
void USART1_SendChar(uint8_t ch);
void USART1_SendString(uint8_t *str);
uint8_t IR_Received = 0;
uint32_t IR_ReceivedData = 0;
int main(void)
{
IR_Decode_Init();
USART1_Config();
while(1)
{
if(IR_Received)
{
USART1_SendString("Received NEC Code: ");
USART1_SendChar((IR_ReceivedData >> 24) & 0xFF);
USART1_SendChar((IR_ReceivedData >> 16) & 0xFF);
USART1_SendChar((IR_ReceivedData >> 8) & 0xFF);
USART1_SendChar(IR_ReceivedData & 0xFF);
USART1_SendString("\r\n");
IR_Received = 0;
}
}
}
void IR_Decode_Init(void)
{
IR_Decode_GPIO_Config();
IR_Decode_EXTI_Config();
}
void IR_Decode_GPIO_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_8;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStruct);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(GPIOB, &GPIO_InitStruct);
}
void IR_Decode_EXTI_Config(void)
{
EXTI_InitTypeDef EXTI_InitStruct;
NVIC_InitTypeDef NVIC_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_AFIO, ENABLE);
EXTI_InitStruct.EXTI_Line = EXTI_Line9;
EXTI_InitStruct.EXTI_Mode = EXTI_Mode_Interrupt;
EXTI_InitStruct.EXTI_Trigger = EXTI_Trigger_Falling;
EXTI_InitStruct.EXTI_LineCmd = ENABLE;
EXTI_Init(&EXTI_InitStruct);
GPIO_EXTILineConfig(GPIO_PortSourceGPIOB, GPIO_PinSource9);
NVIC_InitStruct.NVIC_IRQChannel = EXTI9_5_IRQn;
NVIC_InitStruct.NVIC_IRQChannelPreemptionPriority = 0x00;
NVIC_InitStruct.NVIC_IRQChannelSubPriority = 0x00;
NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStruct);
}
void EXTI9_5_IRQHandler(void)
{
if(EXTI_GetITStatus(EXTI_Line9) != RESET)
{
static uint32_t IR_LastTime = 0;
static uint32_t IR_Data = 0;
static uint8_t IR_BitCount = 0;
uint32_t IR_CurrentTime = TIM2->CNT;
if(IR_CurrentTime - IR_LastTime > 4500 && IR_CurrentTime - IR_LastTime < 5000)
{
IR_Data = 0;
IR_BitCount = 0;
}
else if(IR_CurrentTime - IR_LastTime > 2250 && IR_CurrentTime - IR_LastTime < 2750)
{
IR_Data = (IR_Data << 1) | 0x01;
IR_BitCount++;
if(IR_BitCount == 32)
{
IR_ReceivedData = IR_Data;
IR_Received = 1;
}
}
else if(IR_CurrentTime - IR_LastTime > 560 && IR_CurrentTime - IR_LastTime < 680)
{
IR_Data = (IR_Data << 1) & 0xFFFFFFFE;
IR_BitCount++;
if(IR_BitCount == 32)
{
IR_ReceivedData = IR_Data;
IR_Received = 1;
}
}
IR_LastTime = IR_CurrentTime;
EXTI_ClearITPendingBit(EXTI_Line9);
}
}
void USART1_Config(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
USART_InitTypeDef USART_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStruct);
USART_InitStruct.USART_BaudRate = 115200;
USART_InitStruct.USART_WordLength = USART_WordLength_8b;
USART_InitStruct.USART_StopBits = USART_StopBits_1;
USART_InitStruct.USART_Parity = USART_Parity_No;
USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStruct.USART_Mode = USART_Mode_Tx;
USART_Init(USART1, &USART_InitStruct);
USART_Cmd(USART1, ENABLE);
}
void USART1_SendChar(uint8_t ch)
{
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
USART_SendData(USART1, ch);
}
void USART1_SendString(uint8_t *str)
{
while(*str)
{
USART1_SendChar(*str++);
}
}
```
这段程序的实现过程是:首先通过PB9引脚连接一个红外接收头,然后当接收到红外信号时,通过EXTI中断进行解码,将解出的码存储在IR_ReceivedData变量中。程序通过USART1串口将解码出的码发送出去,使用PB8引脚连接一个红外发射器即可实现发送功能。
阅读全文
相关推荐
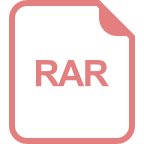
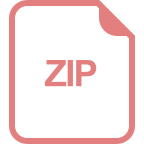
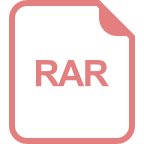
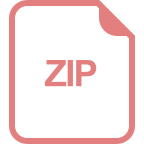
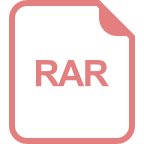
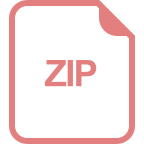
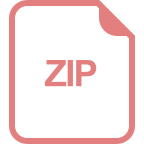
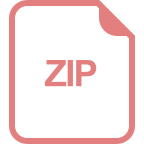
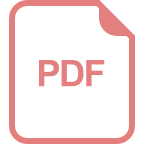
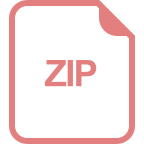
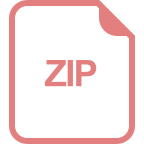
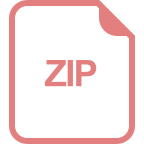
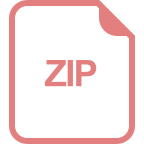
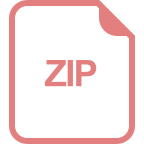
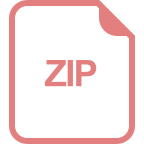
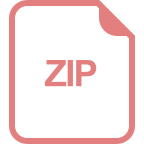
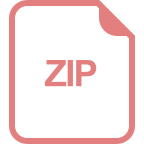