返回字符串 “code” 在给定字符串中任意位置出现的次数,接受 'd' 的任何字母,用python怎么写
时间: 2024-09-23 15:15:58 浏览: 87
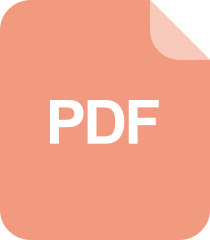
python统计字符串中指定字符出现次数的方法

在Python中,你可以使用内置的`str.count()`函数来计算特定字符或子串在字符串中出现的次数。假设你想查找字符串 "code" 在输入字符串中出现的次数,不管 "c", "o", 或 "d" 是大写还是小写,可以这样做:
```python
def count_substring(input_string, substring):
# 将输入字符串和子串都转换为小写,以便不区分大小写比较
input_string = input_string.lower()
substring = substring.lower()
return input_string.count(substring)
# 示例用法
input_string = "This is a Code sample with multiple codEs"
substring = "code"
print(count_substring(input_string, substring))
```
在这个例子中,`count_substring`函数接收两个参数:一个是输入字符串,另一个是你想要计数的子串。函数先将两者转成小写,然后使用`str.count()`返回子串在输入字符串中出现的次数。
阅读全文
相关推荐
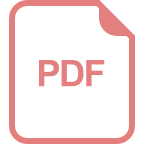
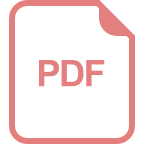

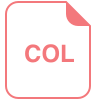












