修改这段代码里的错误 不能修改animal类 接口 main函数 import java.util.Scanner; public class Main { public static void main(String[] args) { Scanner input=new Scanner(System.in); IAbility animal=null; int type=input.nextInt(); String name=input.next(); int age=input.nextInt(); if (type==1) animal=new Dog(name,age); else animal=new Cat(name,age); Simulator sim=new Simulator(); sim.playSound(animal); input.close(); } } abstract class Animal{ private String name; //名字 private int age; //年龄 public Animal(String name, int age) { this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } } interface IAbility{ void showInfo(); //输出动物信息 void cry(); //动物发出叫声 } class Dog extends Animal implements IAbility { Dog(String name, int age) { super(name,age); } public void showInfo() { System.out.println("我是一只狗,我的名字是" + super.getName() + ",今年 " + super.getAge() +"岁"); } public void cry() { System.out.println("旺旺"); } } class Cat extends Animal implements IAbility { Cat(String name, int age) { super(name,age); } public void showInfo() { System.out.println("我是一只猫,我的名字是" + super.getName() + ",今年 " + super.getAge() +"岁"); } public void cry() { System.out.println("喵喵"); } } class Simulator { public void playSound(IAbility animal) { animal.showInfo(); animal.cry(); System.out.println(animal.getName()); } }
时间: 2024-03-16 18:42:34 浏览: 66
好的,这段代码存在两个错误:
1. 在 `Simulator` 类的 `playSound` 方法中,最后一个 `println` 语句中的 `name` 应该改为 `getName()` 方法调用。
2. 在 `Dog` 和 `Cat` 类中,构造方法的访问权限应该改为 `public`。
以下是修改后的代码:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
IAbility animal = null;
int type = input.nextInt();
String name = input.next();
int age = input.nextInt();
if (type == 1) {
animal = new Dog(name, age);
} else {
animal = new Cat(name, age);
}
Simulator sim = new Simulator();
sim.playSound(animal);
input.close();
}
}
abstract class Animal {
private String name; // 名字
private int age; // 年龄
public Animal(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
interface IAbility {
void showInfo(); // 输出动物信息
void cry(); // 动物发出叫声
}
class Dog extends Animal implements IAbility {
public Dog(String name, int age) {
super(name, age);
}
public void showInfo() {
System.out.println("我是一只狗,我的名字是" + super.getName() + ",今年 " + super.getAge() + "岁");
}
public void cry() {
System.out.println("旺旺");
}
}
class Cat extends Animal implements IAbility {
public Cat(String name, int age) {
super(name, age);
}
public void showInfo() {
System.out.println("我是一只猫,我的名字是" + super.getName() + ",今年 " + super.getAge() + "岁");
}
public void cry() {
System.out.println("喵喵");
}
}
class Simulator {
public void playSound(IAbility animal) {
animal.showInfo();
animal.cry();
System.out.println(animal.getName());
}
}
```
阅读全文
相关推荐
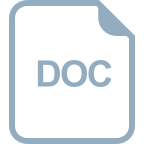
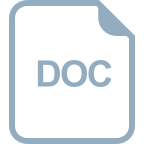
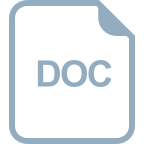










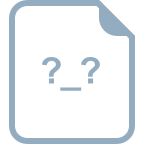





