plot2() { const limit2 = this.tableData.length; let y0 = []; let x0 = []; for (let i = 0; i <= limit2 - 1; i++) { y0.push(parseFloat(this.tableData[i].flux)); x0.push(parseFloat(this.tableData[i].date)); } console.log(y0) console.log(x0) let z1 = []; for (let i = 0; i < x0.length; i++) z1.push([x0[i],y0[i]]); charts = echarts.init(document.getElementById('chart2')); let option2 = { title: { text: '涌水量-时间' }, tooltip: { trigger: 'axis', axisPointer: { type: 'cross' } }, yAxis: { type: 'value', name: '涌水量' }, xAxis: { type: 'time', // 自定义时间格式 axisLabel: { formatter: function (value) { return echarts.format.formatTime('yyyy-MM-dd', value); } }, name: '时间' }, series: [{ data: z1, type: 'line', name: '涌水量', yAxisIndex: 0 }] }, charts.setOption(option2) }这段代码如何修改
时间: 2024-01-12 10:03:15 浏览: 123
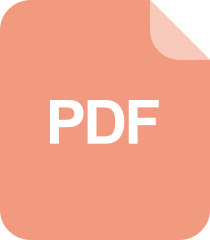
matplotilb中fig,ax = plt.subplots()的使用
这段代码可能需要进行以下修改:
1. 变量`charts`需要使用`let`或`const`关键字进行声明。
2. 函数`plot2()`可能需要传入参数,或者使用`this`关键字来引用当前作用域中的变量。
3. 在`x0`和`y0`数组的填充过程中,需要进行有效性检查,例如判断`this.tableData[i]`是否存在,以及对`parseFloat()`的返回值进行检查。
4. 在`option2`对象的定义中,需要对`series`属性进行更详细的配置,以便正确地呈现数据。例如,可以添加`smooth: true`来设置折线图的平滑程度。
改进后的代码示例如下:
```javascript
function plot2(tableData) {
const limit2 = tableData.length;
let y0 = [];
let x0 = [];
// 进行有效性检查,并将数据解析为数值类型
for (let i = 0; i < limit2; i++) {
if (tableData[i] && tableData[i].flux && tableData[i].date) {
y0.push(parseFloat(tableData[i].flux));
x0.push(new Date(tableData[i].date));
}
}
console.log(y0);
console.log(x0);
let z1 = [];
// 将数据转换为 [x, y] 的形式
for (let i = 0; i < x0.length; i++) {
z1.push([x0[i], y0[i]]);
}
// 使用 let 或 const 声明变量
const charts = echarts.init(document.getElementById('chart2'));
const option2 = {
title: {
text: '涌水量-时间',
},
tooltip: {
trigger: 'axis',
axisPointer: {
type: 'cross',
},
},
yAxis: {
type: 'value',
name: '涌水量',
},
xAxis: {
type: 'time',
axisLabel: {
formatter: function (value) {
return echarts.format.formatTime('yyyy-MM-dd', value);
},
},
name: '时间',
},
series: [
{
// 添加 smooth: true 来设置折线图的平滑程度
data: z1,
type: 'line',
name: '涌水量',
yAxisIndex: 0,
smooth: true,
},
],
};
charts.setOption(option2);
}
```
注意,这只是对代码进行了一些基本的修改,并不能保证在具体应用场景中一定能够正常运行。在实际开发中,需要根据具体情况进行调试和优化。
阅读全文
相关推荐
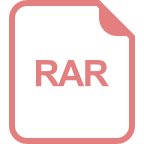
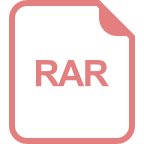

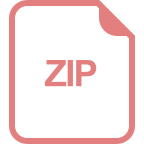
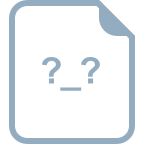
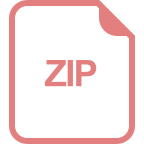
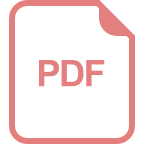
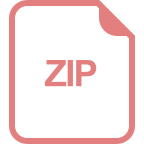
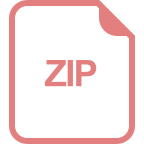
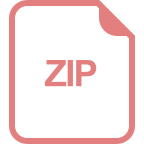
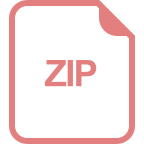
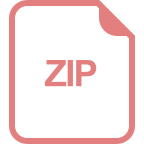
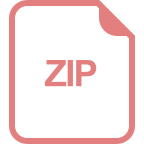
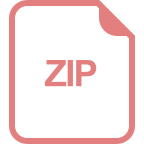
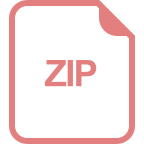
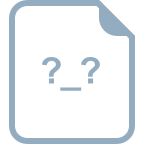
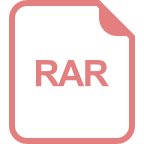