beginbatchdraw();的用法
时间: 2024-04-09 20:31:10 浏览: 121
beginbatchdraw()是一个函数,用于在绘图窗口中开始批量绘制图形。在调用该函数后,所有的绘图操作都将被缓存起来,直到调用endbatchdraw()函数时才会一次性绘制出来,从而提高绘图效率。在使用beginbatchdraw()函数时,需要注意不要在绘图操作期间进行其他的操作,否则可能会导致绘图出现异常。
相关问题
easyx双缓冲绘图 void BeginBatchDraw(); void FlushBatchDraw()怎么用
EasyX提供了双缓冲绘图的两个函数BeginBatchDraw()和FlushBatchDraw(),使用这两个函数可以简化双缓冲绘图的实现过程。
BeginBatchDraw()函数用于开启批量绘图模式,该模式下所有的绘图操作都被缓存到内存中,不会立即显示在屏幕上。
FlushBatchDraw()函数用于将缓存的绘图操作刷新到屏幕上,从而显示出来。
具体使用方法如下:
```c++
#include <graphics.h>
int main()
{
initgraph(640, 480); // 初始化图形界面
BeginBatchDraw(); // 开启批量绘图模式
// 在缓存中进行绘图操作
setbkcolor(WHITE);
cleardevice();
settextcolor(BLACK);
outtextxy(100, 100, "Hello, world!");
FlushBatchDraw(); // 将缓存中的绘图操作刷新到屏幕上
// 等待用户按下任意键
getch();
closegraph(); // 关闭图形界面
return 0;
}
```
在上面的代码中,我们使用BeginBatchDraw()函数开启批量绘图模式,然后在缓存中进行绘图操作,最后使用FlushBatchDraw()函数将缓存中的绘图操作刷新到屏幕上。注意,在使用BeginBatchDraw()和FlushBatchDraw()函数时,不需要使用setvisualpage()和setactivepage()函数进行页面的切换,EasyX会自动帮我们完成这个过程。
使用BeginBatchDraw()和FlushBatchDraw()函数可以让双缓冲绘图的实现更加简单和方便。
优化这段程序:#include<amp_graphics.h> #include<conio.h> #include<vector> using namespace std; #define WIDTH 1000 #define HEIGHT 700 class Point {//定义顶点类坐标 public: float x, y; Point(float ix, float iy) { x = ix; y = iy; } }; class Path{ public: vector<Point>keyPoints; void draw() { setlinecolor(RGB(0, 0, 0)); setfillcolor(RGB(0, 0, 0)); for (int i = 0;i<keyPoints.size(); i++) { fillcircle(keyPoints[i].x, keyPoints[i].y, 8); } for (int i = 0; i < keyPoints.size(); i++) { line(keyPoints[i].x, keyPoints[i].y, keyPoints[i+1].x, keyPoints[i+1].y); } } }; Path path; void startup() { initgraph(WIDTH, HEIGHT); setbkcolor(WHITE); cleardevice(); path.keyPoints.push_back(Point(100, 600)); path.keyPoints.push_back(Point(900, 600)); path.keyPoints.push_back(Point(900, 100)); path.keyPoints.push_back(Point(100, 100)); BeginBatchDraw(); } void show() { cleardevice(); path.draw(); FlushBatchDraw(); sleep(10); } int main() { startup(); while (1) { show(); } return 0; }
以下是对代码的一些优化建议:
1. 优化图形库的引入方式:`#include <amp_graphics.h>` 和 `#include <conio.h>` 这两个头文件可能是错误的或者不存在,如果确实需要图形库,请使用正确的头文件引入方式。
2. 减少命名空间的使用:避免在全局范围内使用 `using namespace std;`,这可能会导致命名冲突,可以直接在使用标准库的地方加上 `std::` 前缀。
3. 使用常量代替宏定义:可以使用 `const` 关键字定义常量,代替宏定义 `#define`。
4. 避免使用无限循环:可以考虑使用条件语句来控制程序的运行,避免无限循环。
5. 优化路径绘制:在 `Path` 类的 `draw` 方法中,可以考虑遍历 `keyPoints` 容器时使用迭代器,以提高性能和代码的简洁性。同时,在绘制直线时,需要注意最后一个点与第一个点的连接。
下面是优化后的代码示例:
```cpp
#include <graphics.h>
#include <vector>
const int WIDTH = 1000;
const int HEIGHT = 700;
class Point {
public:
float x, y;
Point(float ix, float iy) {
x = ix;
y = iy;
}
};
class Path {
public:
std::vector<Point> keyPoints;
void draw() {
setlinecolor(RGB(0, 0, 0));
setfillcolor(RGB(0, 0, 0));
for (auto it = keyPoints.begin(); it != keyPoints.end(); ++it) {
fillcircle(it->x, it->y, 8);
}
for (size_t i = 0; i < keyPoints.size() - 1; ++i) {
line(keyPoints[i].x, keyPoints[i].y, keyPoints[i + 1].x, keyPoints[i + 1].y);
}
// 最后一个点与第一个点的连接
line(keyPoints.back().x, keyPoints.back().y, keyPoints.front().x, keyPoints.front().y);
}
};
int main() {
initgraph(WIDTH, HEIGHT);
setbkcolor(WHITE);
cleardevice();
Path path;
path.keyPoints.push_back(Point(100, 600));
path.keyPoints.push_back(Point(900, 600));
path.keyPoints.push_back(Point(900, 100));
path.keyPoints.push_back(Point(100, 100));
while (!kbhit()) {
cleardevice();
path.draw();
FlushBatchDraw();
Sleep(10);
}
closegraph();
return 0;
}
```
这些优化将提高代码的可读性和性能,并修复了一些潜在的问题。请注意,这段代码使用了 EasyX 图形库,请确保已经正确安装并配置了该库才能运行。
阅读全文
相关推荐
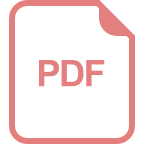
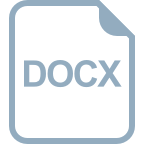
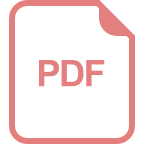
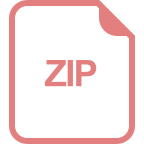
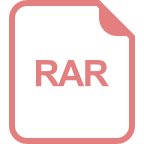
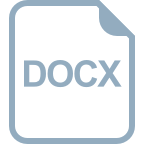
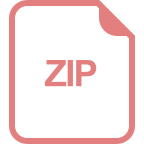
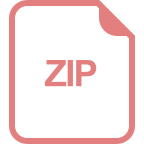
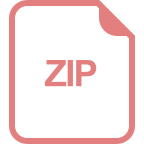
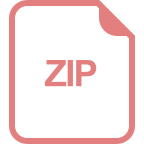
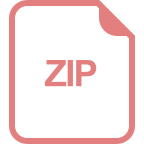