java 根据正则生成数据
时间: 2023-10-12 07:05:10 浏览: 142
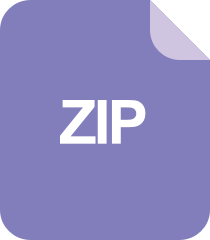
java Xeger 根据正则表达式生成满足的随机数据
Java 中可以使用正则表达式来生成数据,具体实现方式如下:
1. 定义正则表达式,例如:
```
String regex = "[a-zA-Z0-9]{8}";
```
该正则表示生成长度为 8 的由字母和数字组成的字符串。
2. 使用 Random 类生成随机字符串,例如:
```
Random random = new Random();
String result = "";
while (result.length() < 8) {
result += (char) (random.nextInt(26) + 'a');
}
```
该代码片段使用 Random 类生成随机字符,并将其拼接成字符串。
3. 使用 Matcher 类进行正则匹配,例如:
```
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(result);
if (matcher.matches()) {
System.out.println(result);
}
```
该代码片段使用 Pattern 类将正则表达式编译成模式,然后使用 Matcher 类对随机生成的字符串进行匹配。如果匹配成功,则输出该字符串。
完整代码示例:
```
import java.util.Random;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Main {
public static void main(String[] args) {
String regex = "[a-zA-Z0-9]{8}";
Random random = new Random();
String result = "";
while (result.length() < 8) {
result += (char) (random.nextInt(26) + 'a');
}
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(result);
if (matcher.matches()) {
System.out.println(result);
}
}
}
```
阅读全文
相关推荐
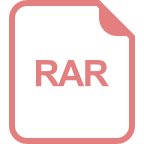















