c语言生成json文件
时间: 2023-12-11 21:02:00 浏览: 27
要在C语言中生成JSON文件,您可以使用一个叫做 cJSON 的开源库。cJSON是一个轻量级的JSON解析/生成库,可用于C中的嵌入式系统。
以下是一个生成JSON文件的示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include "cJSON.h"
int main() {
cJSON *root = NULL;
cJSON *array = NULL;
cJSON *item = NULL;
char *json_string = NULL;
// 创建JSON对象
root = cJSON_CreateObject();
if (root == NULL) {
goto end;
}
// 添加JSON数组
array = cJSON_AddArrayToObject(root, "my_array");
if (array == NULL) {
goto end;
}
// 添加数组元素
item = cJSON_CreateNumber(100);
if (item == NULL) {
goto end;
}
cJSON_AddItemToArray(array, item);
item = cJSON_CreateNumber(200);
if (item == NULL) {
goto end;
}
cJSON_AddItemToArray(array, item);
// 生成JSON字符串
json_string = cJSON_Print(root);
if (json_string == NULL) {
goto end;
}
// 输出JSON字符串
printf("%s\n", json_string);
end:
// 释放内存
if (root != NULL) {
cJSON_Delete(root);
}
if (json_string != NULL) {
free(json_string);
}
return 0;
}
```
在这个示例程序中,我们首先创建了一个JSON对象(使用 `cJSON_CreateObject()` 函数)。然后,我们添加了一个名为“my_array”的数组到JSON对象中(使用 `cJSON_AddArrayToObject()` 函数)。接下来,我们添加了两个数组元素(使用 `cJSON_CreateNumber()` 函数和 `cJSON_AddItemToArray()` 函数)。
最后,我们使用 `cJSON_Print()` 函数生成JSON字符串,并输出它。注意,我们必须使用 `free()` 函数释放 `cJSON_Print()` 函数返回的字符串。
这只是一个简单的示例,cJSON库还有很多其他功能,例如解析JSON字符串等。您可以查阅cJSON库的文档,以获得更多信息。
相关推荐
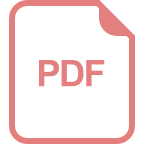














