Java 多个条形码生成,返回前台预览,并可选打印机打印
时间: 2023-08-16 18:06:34 浏览: 195
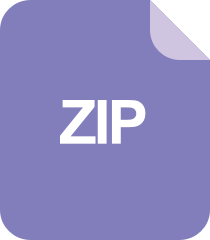
java实现斑马打印机 ZPL指令打印条码.zip
您可以使用一些 Java 的条形码生成库(如Zxing、Barbecue等)来生成多个条形码图片,并将这些图片返回给前台进行预览和打印。以下是一个简单的实现示例:
```java
import java.awt.image.BufferedImage;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.imageio.ImageIO;
import javax.servlet.http.HttpServletResponse;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.WriterException;
import com.google.zxing.client.j2se.MatrixToImageWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
@Controller
@RequestMapping("/barcode")
public class BarcodeController {
@GetMapping("/generate")
@ResponseBody
public List<String> generateBarcodes(@RequestParam("data") List<String> data,
HttpServletResponse response) throws IOException {
List<String> barcodeUrls = new ArrayList<>();
for (String d : data) {
// 生成条形码
BufferedImage barcodeImage = generateBarcode(d);
// 将图片转换为BASE64编码字符串
String barcodeUrl = imageToBase64(barcodeImage);
barcodeUrls.add(barcodeUrl);
}
return barcodeUrls;
}
private BufferedImage generateBarcode(String data) {
int width = 300;
int height = 100;
String format = "png";
BarcodeFormat barcodeFormat = BarcodeFormat.CODE_128;
ErrorCorrectionLevel errorCorrectionLevel = ErrorCorrectionLevel.L;
// 设置条形码参数
com.google.zxing.EncodeHintType hintType = EncodeHintType.ERROR_CORRECTION;
com.google.zxing.EncodeHintType encodingType = EncodeHintType.CHARACTER_SET;
com.google.zxing.EncodeHintType marginType = EncodeHintType.MARGIN;
int margin = 2;
java.util.Map<com.google.zxing.EncodeHintType, Object> hints = new java.util.HashMap<>();
hints.put(hintType, errorCorrectionLevel);
hints.put(encodingType, "UTF-8");
hints.put(marginType, margin);
BitMatrix bitMatrix = null;
try {
bitMatrix = new com.google.zxing.MultiFormatWriter().encode(data, barcodeFormat, width, height, hints);
} catch (WriterException e) {
e.printStackTrace();
}
BufferedImage image = MatrixToImageWriter.toBufferedImage(bitMatrix);
return image;
}
private String imageToBase64(BufferedImage image) throws IOException {
String base64 = null;
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
try {
ImageIO.write(image, "png", outputStream);
byte[] bytes = outputStream.toByteArray();
base64 = java.util.Base64.getEncoder().encodeToString(bytes);
} finally {
outputStream.close();
}
return base64;
}
}
```
在上面的示例中,我们使用了Zxing库生成条形码,将生成的条形码图片转换为BASE64编码字符串,并将多个条形码的URL返回给前台。您可以使用JavaScript等前端工具将这些URL转换为图片进行预览和打印。
阅读全文
相关推荐
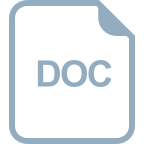
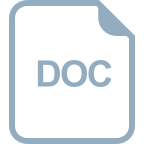
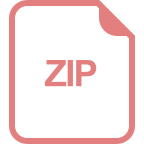
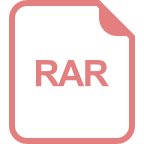
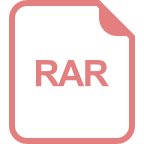
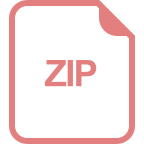
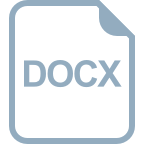
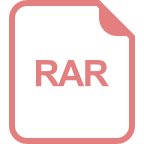
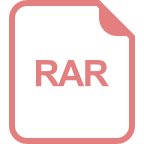
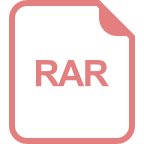
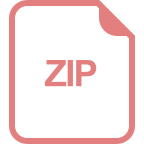
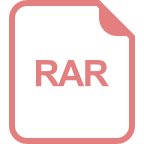
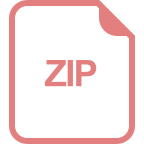
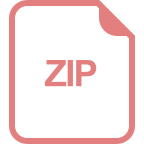