priorityqueue有pop_back
时间: 2023-11-22 18:48:42 浏览: 130
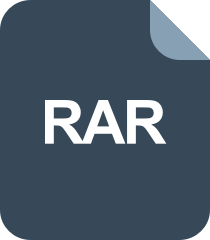
priorityqueue.rar_Applet_Java_
根据提供的引用内容,priority_queue是一个基于优先级的队列,它的主要作用是在程序中按照优先级对元素进行排序。在priority_queue中,元素的插入和删除都是按照优先级进行的,因此它不支持pop_back操作。priority_queue只支持pop和push操作,其中pop操作会删除队列中优先级最高的元素,而push操作会将新元素插入到队列中并按照优先级进行排序。
阅读全文
相关推荐
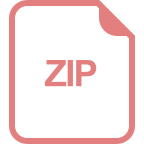
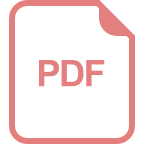
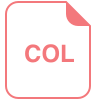
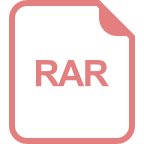
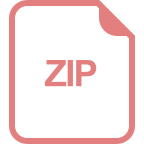
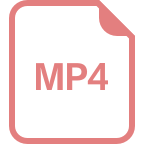
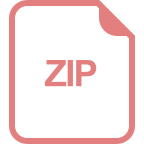
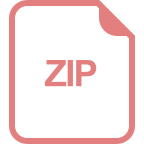
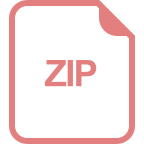
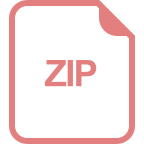