c++单链表实现通讯录橙意
时间: 2024-05-16 17:09:27 浏览: 92
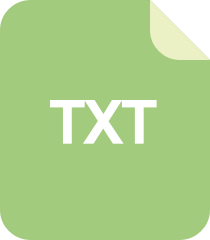
C++中用链表实现的通讯录

C++单链表可以用来实现通讯录功能,下面是一个简单的示例:
```cpp
#include <iostream>
using namespace std;
// 定义通讯录节点结构
struct ContactNode {
string name;
string phoneNumber;
ContactNode* next;
};
// 添加联系人
void addContact(ContactNode*& head, string name, string phoneNumber) {
// 创建新节点
ContactNode* newNode = new ContactNode;
newNode->name = name;
newNode->phoneNumber = phoneNumber;
newNode->next = nullptr;
// 如果链表为空,将新节点设为头节点
if (head == nullptr) {
head = newNode;
}
else {
// 找到链表末尾节点
ContactNode* current = head;
while (current->next != nullptr) {
current = current->next;
}
// 将新节点添加到末尾
current->next = newNode;
}
}
// 显示通讯录
void displayContacts(ContactNode* head) {
ContactNode* current = head;
while (current != nullptr) {
cout << "Name: " << current->name << ", Phone Number: " << current->phoneNumber << endl;
current = current->next;
}
}
int main() {
ContactNode* head = nullptr;
// 添加联系人
addContact(head, "Alice", "123456789");
addContact(head, "Bob", "987654321");
addContact(head, "Charlie", "456789123");
// 显示通讯录
displayContacts(head);
return 0;
}
```
这个示例中,我们定义了一个`ContactNode`结构体,包含姓名、电话号码和指向下一个节点的指针。然后,我们实现了两个函数:`addContact`用于添加联系人,`displayContacts`用于显示通讯录中的所有联系人。
阅读全文
相关推荐
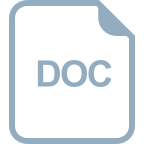
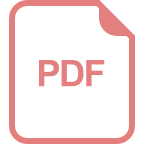
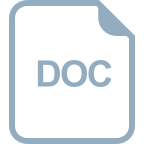
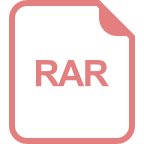
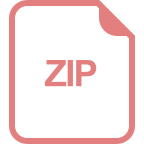
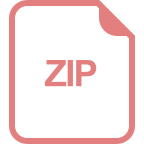
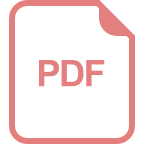
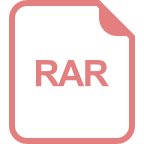
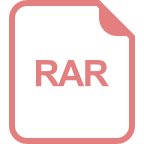
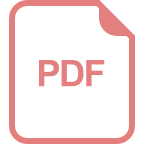
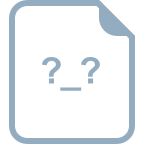
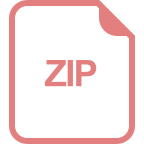
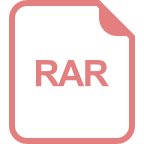
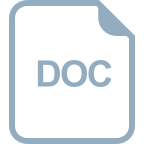