C语言多线程代码示例,要求在Dev-c++中能运行
时间: 2024-09-23 13:11:43 浏览: 41
在C语言中,多线程通常通过POSIX线程库(pthreads)来实现。这里有一个简单的多线程示例,它创建了两个线程,分别打印数字:
```c
#include <stdio.h>
#include <pthread.h>
// 定义线程函数
void* print_numbers(void* arg) {
long num = (long)arg;
for (int i = 0; i < 5; i++) {
printf("Thread %ld: %d\n", (long)pthread_self(), num++);
sleep(1); // 暂停一秒以模拟工作
}
return NULL;
}
int main() {
pthread_t thread1, thread2; // 创建线程的句柄
int rc;
// 创建并启动线程1,打印1到5
rc = pthread_create(&thread1, NULL, print_numbers, (void*)1);
if (rc) {
perror("Error creating thread 1");
return 1;
}
// 创建并启动线程2,打印6到10
rc = pthread_create(&thread2, NULL, print_numbers, (void*)6);
if (rc) {
perror("Error creating thread 2");
return 1;
}
// 等待两个线程结束
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
printf("Main thread completed.\n");
return 0;
}
```
在这个例子中,`print_numbers`是一个线程函数,接受一个整数作为参数。`main`函数首先创建两个线程,然后等待它们执行完毕。
要在Dev-C++中编译并运行这个程序,你需要包含pthread头文件,并链接`-lpthread`库。例如,在项目的项目属性设置里,点击"Settings" -> "Linker" -> "Other Linker Options",添加 `-lpthread`。
阅读全文
相关推荐
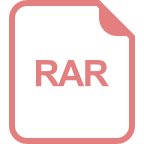
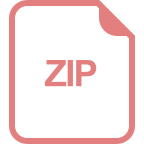















