cannot import name 'decimal' from 'decimal'
时间: 2023-10-11 07:08:09 浏览: 33
当出现"cannot import name 'decimal' from 'decimal'"的错误时,通常是因为引用了不存在的模块。根据你提供的引用内容,错误可能是由于你拼写错误导致的。在第一个引用中,你导入了decimal模块并使用了它的Decimal类,但是你拼写了Deciaml而不是Decimal。请检查你的代码并确保正确导入和拼写decimal模块及其类名。
相关问题
from decimal import是什么意思
`from decimal import` 是 Python 中的一条导入语句,它的作用是从 decimal 模块中导入所有的函数、类和常量,以便在代码中直接使用它们,而不需要使用完整的模块名。在上面的代码中,我们使用了 Decimal 类型来实现高精度计算,这个类型是在 decimal 模块中定义的。因此,我们需要使用 `from decimal import *` 来导入这个模块中的所有内容,以便在代码中直接使用 Decimal 类型。如果没有使用这条导入语句,我们在代码中使用 Decimal 类型时需要写成 `decimal.Decimal` 的形式。
from flask import Flask,request,render_template,jsonify from flask_cors import cross_origin,CORS import pymysql from pymysql import cursors from decimal import *解释每行代码意思
- `from flask import Flask,request,render_template,jsonify`: 这行代码导入了 Flask 框架中的 Flask、request、render_template 和 jsonify 模块。Flask 是一个 Python web 框架,request 模块用于处理 HTTP 请求,render_template 模块用于渲染 HTML 模板,jsonify 模块用于将 Python 对象转换为 JSON 格式。
- `from flask_cors import cross_origin,CORS`: 这行代码导入了 Flask-CORS 模块中的 cross_origin 和 CORS 模块。Flask-CORS 是一个 Flask 扩展,可以解决跨域请求的问题。
- `import pymysql`: 这行代码导入了 PyMySQL 模块,PyMySQL 是一个 Python MySQL 库,可以用于连接和操作 MySQL 数据库。
- `from pymysql import cursors`: 这行代码导入了 PyMySQL 模块中的 cursors 模块,cursors 模块提供了游标操作的功能。
- `from decimal import *`: 这行代码导入了 Python 内置的 decimal 模块,decimal 模块用于高精度计算。在一些需要精确计算的场景中,使用 decimal 模块可以避免浮点数计算带来的精度误差问题。
相关推荐
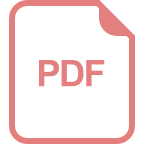
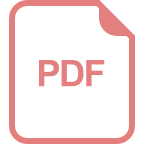












