C++读写json文件
时间: 2024-09-24 16:11:56 浏览: 39
在C++中读写JSON文件通常会使用第三方库,因为C++标准库本身并不直接支持。两个常用的库是RapidJSON和nlohmann/json。以下是使用这两个库的基本示例:
1. **RapidJSON** (https://github.com/miloyip/rapidjson):
```cpp
#include "rapidjson/document.h"
#include "rapidjson/writer.h"
#include "fstream"
void writeJson(const rapidjson::Value& value, const std::string& filename) {
rapidjson::FileWriteStream out(filename);
rapidjson::PrettyWriter<rapidjson::FileWriteStream> writer(out);
writer.writeDocument(value);
}
void readJson(const std::string& filename, rapidjson::Value& value) {
std::ifstream in(filename);
if (!in) {
// 错误处理...
return;
}
rapidjson::StreamReadParser parser(in);
value.ParseStream(parser);
}
```
2. **nlohmann/json** (https://github.com/nlohmann/json):
```cpp
#include <nlohmann/json.hpp>
using json = nlohmann::json;
void writeJson(const json& j, const std::string& filename) {
std::ofstream file(filename);
file << j.dump(4); // 第四个参数控制缩进,便于阅读
}
void readJson(const std::string& filename, json& j) {
std::ifstream input(filename);
input >> j;
if (!input) {
// 错误处理...
}
}
```
在使用之前,请确保已安装并链接相应的库到你的项目中。
阅读全文
相关推荐
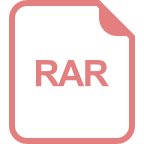
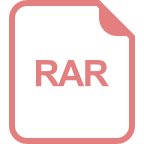
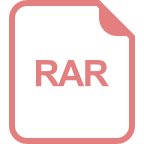















