几何,假设平面上有任意三角形,编写程序,提示用户输入一个点坐标的x,y坐标,然后判断这个点是否在三角形内。用java写
时间: 2024-09-25 22:04:50 浏览: 97
在Java中,判断一个点是否位于给定的三角形内部可以使用向量的方法来实现。首先,我们需要确定三个顶点A、B、C的坐标,然后计算出从每个顶点到该点的向量,并检查它们是否满足“右手规则”或“叉乘法”来确定位置。
以下是基本步骤的伪代码:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// 获取三角形顶点坐标
System.out.println("请输入三角形第一个顶点A的坐标 (x1, y1):");
double x1 = scanner.nextDouble();
double y1 = scanner.nextDouble();
double x2 = scanner.nextDouble(); // 假设第二个顶点B的坐标
double y2 = scanner.nextDouble();
double x3 = scanner.nextDouble(); // 第三个顶点C的坐标
double y3 = scanner.nextDouble();
// 用户输入新点
System.out.println("请输入待判断点的坐标 (px, py):");
double px = scanner.nextDouble();
double py = scanner.nextDouble();
// 计算向量
Vector AB = new Vector(x2 - x1, y2 - y1);
Vector AC = new Vector(x3 - x1, y3 - y1);
Vector AP = new Vector(px - x1, py - y1);
// 判断点是否在三角形内
if ((AP.crossProduct(AB).sign() > 0 && AP.crossProduct(AC).sign() < 0) ||
(AP.crossProduct(AC).sign() > 0 && AP.crossProduct(AB).sign() < 0)) {
System.out.println("点在三角形内部.");
} else {
System.out.println("点不在三角形内部.");
}
scanner.close();
}
}
class Vector {
double x, y;
// 向量运算方法
Vector crossProduct(Vector other) {
return new Vector(y * other.x - x * other.y, x * other.y - y * other.x);
}
// 签名方法判断向量方向
int sign() {
return this.x >= 0 ? (this.y >= 0 ? 1 : -1) : (this.y >= 0 ? -1 : 1);
}
}
```
在这个例子中,我们创建了一个`Vector`类来处理向量操作,包括交叉积(用于判断方向)和签名校验。注意,这个方法仅适用于逆时针顺序定义的三角形(即角度小于180度),如果是其他情况,需要调整判断条件。
阅读全文
相关推荐
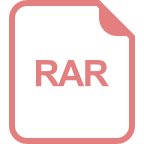
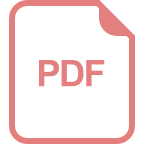
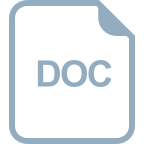
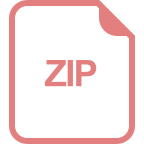
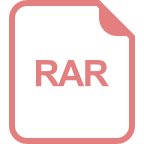
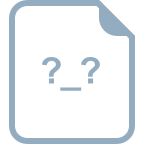
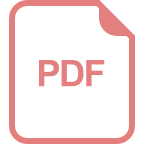
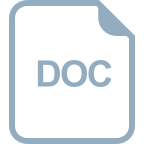
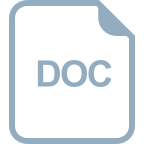
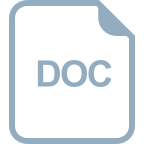
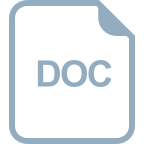
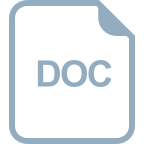
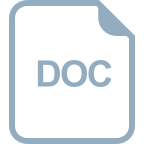
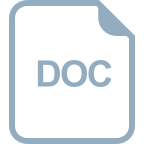
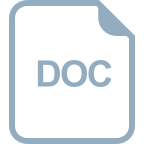
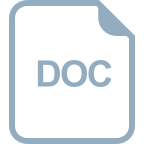
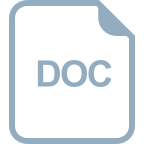
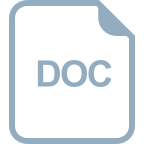
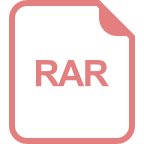