c语言:将一个带头结点的单链表A分解为两个带头结点的单链表B和C,使得B表中含有原表中序号为奇数的元素,而C中含有原表中序号为偶数的元素,且保持其相对顺序不变。(可写伪代码)简单一点
时间: 2024-10-01 08:02:29 浏览: 51
在C语言中,你可以通过遍历链表并根据节点索引的奇偶性来划分成两个链表。这里是一个简单的伪代码描述:
```cpp
// 假设head是指向链表头的指针,每个节点结构体包含val(值)和next(下一个节点)
struct Node {
int val;
struct Node* next;
};
void splitLinkedList(struct Node* head, struct Node** B, struct Node** C) {
struct Node* current = head; // 当前节点
*B = head; // 初始化B链表的头为原链表头
*C = NULL; // 初始化C链表为空
while (current != NULL) {
if (current->val % 2 == 0) { // 如果当前节点值为偶数
if (*C == NULL) { // 若C链表为空,直接添加
*C = current;
} else {
// 否则,找到C链表的最后一个偶数节点并插入
struct Node* temp = *C;
while (temp->next != NULL && temp->next->val % 2 != 0) {
temp = temp->next;
}
temp->next = current;
}
} else { // 如果当前节点值为奇数
if (*B == NULL || current->val > (*B)->val) { // 判断是否需要插入到B链表头部
*B = current;
} else {
// 插入到B链表的适当位置,保持原有顺序
struct Node* prev = *B;
while (prev->next != NULL && prev->next->val < current->val) {
prev = prev->next;
}
prev->next = current;
}
}
current = current->next; // 移动到下一个节点
}
}
```
阅读全文
相关推荐
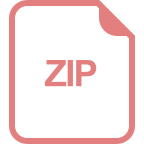
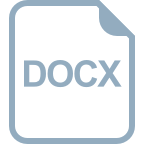
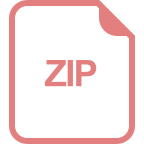






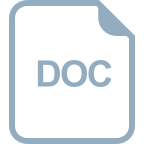

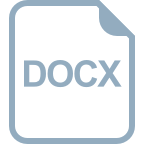
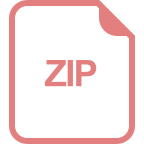
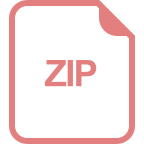
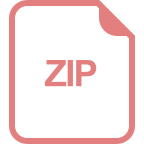
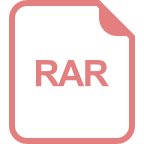
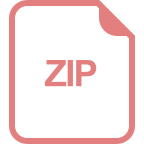