AttributeError: 'Conv3d' object has no attribute 'grad'
时间: 2023-10-30 11:03:17 浏览: 159
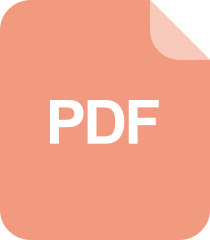
Python3下错误AttributeError: ‘dict’ object has no attribute’iteritems‘的分析与解决

这个错误通常是因为在`Conv3d`对象上使用了`grad`属性,但该属性不存在。可能的原因是在PyTorch版本不匹配的情况下使用了不兼容的函数或方法。解决这个问题的方法有两种:
1. 检查PyTorch的版本:首先,确认你正在使用的PyTorch版本是否支持`grad`属性。可以通过运行以下代码来检查PyTorch版本:
```python
import torch
print(torch.__version__)
```
如果你的PyTorch版本较旧,可能需要升级到与使用的代码相匹配的较新版本。
2. 检查代码:如果你的PyTorch版本已经是兼容的版本,那么错误可能是由于代码中的其他问题引起的。请检查你的代码,确保没有在`Conv3d`对象上使用`grad`属性。可以通过查看错误行的附近代码来定位问题,并根据具体情况进行调整。
请注意,这只是一种可能的解决方案,具体的解决方法可能因你的具体情况而异。如果以上方法不起作用,你可能需要进一步调查或向PyTorch社区寻求帮助。
阅读全文
相关推荐
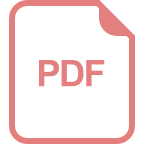
















